How to update columns outside of Editor form scope
How to update columns outside of Editor form scope
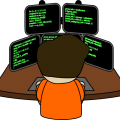
After a bit of struggling I am making some progress with Editor. I haven't yet figured out one important thing though:
How do I update columns in my database table outside of the Editor form scope??
I tried a few things in Javscript but nothing really works. I don't understand how to use "cell()" because I didn't really find a suitable example - and without examples I am lost ...
I also tried something with "preCreate" (see last statement in the code) but it didn't work and I couldn't get hold of the field I wanted to update "userTable.update_id" was wrong ...
This is my Javascript:
function tblUser() {
var userEditor = new $.fn.dataTable.Editor( {
ajax: {
url: 'actions.php?action=tblUser',
type: 'POST',
data: {
lang: lang
}
},
table: "#tblUser",
fields: [ {
label: "Address:",
name: "user.title",
type: "select",
options: [
{ label: "Mr.", value: "Mr." },
{ label: "Ms.", value: "Ms." }
]
}, {
label: "Title (if any):",
name: "user.acad",
type: "select",
options: [
{ label: "-", value: "" },
{ label: "Dr.", value: "Dr." }
]
}, {
label: "First name:",
name: "user.firstname"
}, {
label: "Last name:",
name: "user.lastname"
}, {
label: "Language:",
name: "user.language",
type: "select",
options: [
{ label: "Deutsch", value: "de-DE" },
{ label: "English", value: "en-GB" }
]
}, {
label: "Role:",
name: "user.role",
type: "select",
options: [
{ label: "bla", value: "bla" },
{ label: "blue", value: "blue" }
]
}, {
label: "Email:",
name: "user.email"
}
]
} );
var userTable = $('#tblUser').DataTable( {
dom: "Bfrtip",
ajax: {
url: 'actions.php?action=tblUser',
type: 'POST',
data: {
lang: lang
}
},
columns: [
{ data: null,
render: function ( data, type, row ) {
var titleTransl;
if (lang == 'de') {
if (row.user.title == 'Mr.') {
titleTransl = 'Herr';
} else {
titleTransl = 'Frau';
}
} else {
titleTransl = row.user.title;
}
return titleTransl + ' ' + row.user.acad + ' ' + row.user.firstname + ' ' + row.user.lastname;
}
},
{ data: "user.title" },
{ data: "user.acad" },
{ data: "user.firstname" },
{ data: "user.lastname" },
{ data: "user.language",
render: function ( data, type, row ) {
// return language == 'de-DE' ? 'Deutsch' : 'English';
if (lang == 'de') {
if (row.user.language == 'de-DE') {
return 'Deutsch';
} else {
return 'Englisch';
}
} else {
if (row.user.language == 'de-DE') {
return 'German';
} else {
return 'English';
}
}
}
},
{ data: "user.role" },
{ data: "user.email" },
{ data: "user.updater_id" },
{ data: "user.creator_id" }
],
columnDefs: [
{ targets: [1, 2, 3, 4], visible: false}
],
// lastname and firstname ascending
order: [[ 3, 'asc' ], [ 2, 'asc' ]],
select: {
style: 'single'
},
buttons: [
{ extend: "create", editor: userEditor },
{ extend: "edit", editor: userEditor },
{ extend: "remove", editor: userEditor },
"colvis",
{ extend: "showAll", name: "showAllButton",
action: function ( e, dt, button, config ) {
dt.rows({ selected: false }).nodes().to$().css({"display":"table-row"});
dt.buttons('showAllButton:name').nodes().css({"display":"none"});
//if show all button is clicked, a potential search will be reset and the table is redrawn.
if (dt.search() > '') {
dt.search('').draw();
}
}
}
]
} );
userTable
.on ('select', function ( e, dt, type, indexes) {
dt.rows({ selected: false }).nodes().to$().css({"display":"none"});
dt.buttons('showAllButton:name').nodes().css({"display":"inline", "background-color":"#337AB7", "color":"white"});
});
userTable
.on ('init', function ( e, settings, json) {
userTable.buttons('showAllButton:name').nodes().css({"display":"none"});
});
userTable
.on ('preCreate', function (e, json, data) {
if (userTable.updater_id < 1) {
userTable.updater_id = currentUserId;
}
});
}
And this is my PHP. When I found out I couldn't get the JS working I thought I could play around a little with "getFormatter" and "setFormatter". The get stuff worked; it returned spaces - as desired. But it didn't update the database fields with "$id" - I guess because Editor doesn't know that the fields were updated. PLEASE HELP, thanks.
function tblUser(&$db, &$p, &$lang, &$id) {
if ($lang === 'de') {
$msg[0] = 'Feld darf nicht leer sein.';
$msg[1] = 'Bitte geben Sie eine gültige E-Mail Adresse ein.';
} else {
$msg[0] = 'Field may not be empty.';
$msg[1] = 'Please enter a valid email address.';
}
Editor::inst( $db, 'user' )
->field(
Field::inst( 'user.title' )->validator( 'Validate::notEmpty', array('message' => $msg[0]) ),
Field::inst( 'user.acad' ),
Field::inst( 'user.firstname' )->validator( 'Validate::notEmpty', array('message' => $msg[0]) ),
Field::inst( 'user.lastname' )->validator( 'Validate::notEmpty', array('message' => $msg[0]) ),
Field::inst( 'user.language' )->validator( 'Validate::notEmpty', array('message' => $msg[0]) ),
Field::inst( 'user.role' )->validator( 'Validate::notEmpty', array('message' => $msg[0]) ),
Field::inst( 'user.email' )->validator( 'Validate::email', array(
'required' => true,
'message' => $msg[1]) ),
Field::inst( 'user.updater_id' ) ->getFormatter( function($val, $data, $opts) {
return '';
} )
->setFormatter( 'Format::ifEmpty', $id ),
Field::inst( 'user.creator_id' ) ->getFormatter( function($val, $data, $opts) {
if ($val > 0) {
return $val;
} else {
return '';
}
} )
->setFormatter( 'Format::ifEmpty', $id )
)
->process($p)
->json();
}
This question has accepted answers - jump to:
Answers
Figured out how to do this on the server side which is much better anyway:
I'm not quite clear on the original issue, although from your second post, are you basically looking to find a way of updating a value in the database without sending it from the client-side? If so, the
preCreate
server-side event withsetValue()
is perfect for exactly this sort of thing - nice one.Allan
Thanks Allan. In this case I really don't need to send anything from the client side. So I am fine for now. But would you have an example where you send something from the client side outside the Editor form as well? (I didn't manage to get this done).
As a general comment: Your product is great - but I guess only really advanced developers can use it without the examples (or maybe I am just a below average developer ... I am unable to understand what to do just from reading your code. I need use cases. ). For that reason I would kindly ask you to publish as many examples as possible. Many thanks in advance!
Two possible options depending on how you want things to work.
hidden
fields. The field is in the Editor form, but it just isn't visible to the end user.preSubmit
event handler to get external data from somewhere and add it to the data submitted to the server.Thank you!
Agreed - this is an area that I know I need to improve and hope to do so in 2017. I have some thought about how to go about that such as online courses.
Allan
You made a good point regarding online courses! I restarted coding just this year. I developed my last commercial application in the 1990's ... And that was back end programming with a lot of SQL and programming languages that you've probably never heard of ...
Earlier this year when I got started I benefitted a lot from Udemy.com classes. One of the best and most popular instructors there is a guy named Rob Percival. If you could get a guy like him to develop an online course for your product that could help both you and the instructor. You could refund part of the course fees when someone buys an Editor license etc. to incentivise people a little ...
What makes Rob so unique is not the quality of the content which is ok. It's rather his skills as a teacher. According to Udemy Rob has over 150k people enrolled in his classes.
https://www.udemy.com/user/robpercival/
http://www.businessinsider.de/best-selling-online-classes-udemy-2016-8?r=US&IR=T#/#1-the-complete-web-developer-course-20-1
Awesome - thanks for the links! I'll enjoy watching a few videos from Rob.
Allan