Inserting optgroups into select field
Inserting optgroups into select field
I use this code to add optgroups to a select field's options:
editor
.on('opened', function (e, mode, action) {
$("#DTE_Field_report-report_type_id option").each(function(i) {
if ( i == 0 ) {
$(this).before("<optgroup label='1st group'>");
} else if ( $(this).text().substr(0,2) == "09" ) {
$(this).after("</optgroup><optgroup label='2nd group'>");
} else if ( $(this).text().substr(0,2) == "23" ) {
$(this).after("</optgroup>");
}
});
})
Surprisingly the ending tag </opgroup> is immediately added to the opt group so that it doesn't contain any options. I don't understand why this happens.
It looks like this:
Any idea how to fix this so that the two optgroups actually contain options.
This question has accepted answers - jump to:
This discussion has been closed.
Answers
That's not working because you are attempting to manipulate the DOM like a string there. Line 5 creates a whole
optgroup
for example. Line 7 also creates a singleoptgroup
and line 9 does nothing. You can check that out on the console:What you'd have to do is insert the two optgroups into the
select
and then move theoption
elements that you want into each one.I'd also suggest you do this only once (i.e. immediately after the options have loaded into Editor) rather than on every
opened
- otherwise you might end up adding many option groups!You can't just create new option elements as Editor adds custom properties to them to allow for strict typing of data.
Allan
That sounds very cumbersome. I am loading the options from the server ...
Sure hadn't gotten that far when the question came up
I chose a simple solution because it isn't really required that the options are within an optgroup. All I need is headings for the various groups of options.
This is what I am using now:
optgroups for the select are something I want to add support for in Editor in future! They really are useful for organising data
.
Allan
That would really be useful, Allan!
I modified my solution and really move the options into the optgroups now. That was easier than I thought.
I found this when googling:
The solution above created issues when you do ajax.reloads of the data table. Suddenly you have empty optgroups and stuff like that.
This is now my approach that also works when the table is reloaded from the server without page refresh.
@allan
Could you make those optgroups collapsible as well please.
I have just implemented this using select2.
This is what is looks like uncollapsed:
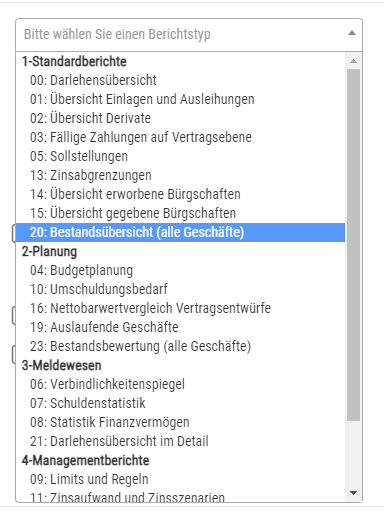
And (partly) collapsed:
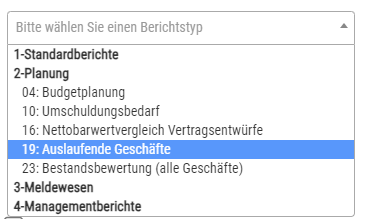
This does the trick when using select2:
I don't believe that is something that is built into the browser. For such a thing you'd need to use Select2 or similar.
Allan
No problem to do that if your select2 plugin allows the definition of outgroups within the Editor api.