Getting the field values from a table row
Getting the field values from a table row
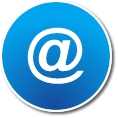
Hi
I am getting confused with getting values from the fields. code example of what I am doing follows
I have taken some of the code from http://datatables.net/forums/discussion/comment/51690
[code]
//
// "Copy" is clicked
//
$(jqDomTableID).on('click', 'a.editor_copy', function(e) {
e.preventDefault();
editor.create('You must change at least one field in order to copy this row.',
{
"label": "Create new row with this data",
"fn": function () { this.submit(); }
});
var fieldlist = editor.fields();
var data = dataTableObject.fnGetData($(this).parents('tr')[0]);
for (var key in data) {
if ($.inArray(key, fieldlist) >= 0) {
editor.set(key, data[key]);
}
else if (data[key].hasOwnProperty('id') && $.inArray(key + '.id', fieldlist) >= 0)
editor.set(key + '.id', data[key].id); // key is the field name, data[key] is the numeric id.
else if ($.inArray(key + '[].id', fieldlist) >= 0) {
var fieldIds = [];
for (var subKey in data[key]) {
if(data[key][subKey].hasOwnProperty('id'))
fieldIds.push(data[key][subKey]['id']);
}
editor.set(key + '[].id', fieldIds);
}
}
});
[/code]
where following line does not retrieve any data but gives me and error
[code]data = dataTableObject.fnGetData($(this).parents('tr')[0]);[/code]
error - TypeError: editor.fnGetData is not a function - on the js debig console
First I thought I had missed some pluggin or similar but I seem to be going round in circles
The final code for testing when I have replace some code just to display an alert with the results
[code]
$('#jobsvisits').on('click', 'a.editor_duplicate', function(e) {
e.preventDefault();
editor.create('Duplicate this row.',
{
"label": "Create new row",
"fn": function () { this.submit(); }
});
var fieldlist = editor.fields();
for (var key in fieldlist) {
alert(editor.get(key)); // This line I have changed many times in order to test
}/**/
});
[/code]
I am getting confused with getting values from the fields. code example of what I am doing follows
I have taken some of the code from http://datatables.net/forums/discussion/comment/51690
[code]
//
// "Copy" is clicked
//
$(jqDomTableID).on('click', 'a.editor_copy', function(e) {
e.preventDefault();
editor.create('You must change at least one field in order to copy this row.',
{
"label": "Create new row with this data",
"fn": function () { this.submit(); }
});
var fieldlist = editor.fields();
var data = dataTableObject.fnGetData($(this).parents('tr')[0]);
for (var key in data) {
if ($.inArray(key, fieldlist) >= 0) {
editor.set(key, data[key]);
}
else if (data[key].hasOwnProperty('id') && $.inArray(key + '.id', fieldlist) >= 0)
editor.set(key + '.id', data[key].id); // key is the field name, data[key] is the numeric id.
else if ($.inArray(key + '[].id', fieldlist) >= 0) {
var fieldIds = [];
for (var subKey in data[key]) {
if(data[key][subKey].hasOwnProperty('id'))
fieldIds.push(data[key][subKey]['id']);
}
editor.set(key + '[].id', fieldIds);
}
}
});
[/code]
where following line does not retrieve any data but gives me and error
[code]data = dataTableObject.fnGetData($(this).parents('tr')[0]);[/code]
error - TypeError: editor.fnGetData is not a function - on the js debig console
First I thought I had missed some pluggin or similar but I seem to be going round in circles
The final code for testing when I have replace some code just to display an alert with the results
[code]
$('#jobsvisits').on('click', 'a.editor_duplicate', function(e) {
e.preventDefault();
editor.create('Duplicate this row.',
{
"label": "Create new row",
"fn": function () { this.submit(); }
});
var fieldlist = editor.fields();
for (var key in fieldlist) {
alert(editor.get(key)); // This line I have changed many times in order to test
}/**/
});
[/code]
This discussion has been closed.
Replies
What value is `dataTableObject` in line 12 of your first example code block? Is it the DataTables instance? Are you able to link me to the page so I can see the full code and see what might be going on?
Thanks,
Allan
Thanks for your reply, my dataTableObject is really called editor as in the second example. I only duplicated the first code from the link on this forum to show the situation I wish to achieve (duplicate a row) .
The page in question is on my localhost so I cant share it but here is the full js code
[code]
(function($){
$(document).ready(function() {
var editor = new $.fn.dataTable.Editor( {
"ajaxUrl": "TableEditor/php/table.jobsvisits.php",
"domTable": "#jobsvisits",
"fields": [
{
"label": "Agent",
"name": "LeadSource",
"type": "select",
"ipOpts":get_jvisits()
},
{
"label": "Visit",
"name": "Visit",
"type": "select",
"ipOpts": [
{"label":"Yes","value":"1"},
{"label":"No","value":"0"}],
"default" : "0"
},
{
"label": "Pack",
"name": "Pack",
"type": "select",
"ipOpts": [
{"label":"none","value":"none"},
{"label":"Full Pack","value":"Full Pack"},
{"label":"MP Pack","value":"MP Pack"},
{"label":"JV Pack","value":"JV Pack"}],
"default" : "none"
}
]
} );
// Edit record
$('#jobsvisits').on('click', 'a.editor_edit', function (e) {
e.preventDefault();
editor.edit(
$(this).parents('tr')[0],
'Edit record',
{ "label": "Update", "fn": function () { editor.submit() } }
);
} );
// Delete a record (without asking a user for confirmation)
$('#jobsvisits').on('click', 'a.editor_remove', function (e) {
e.preventDefault();
editor.message( "Are you sure you want to remove this row?" );
editor.remove( $(this).parents('tr')[0],"Delete Row",{
"label": "Confirm",
"fn": function () { this.submit(); }
},true );
} );
// Duplicate a record (without asking a user for confirmation)
$('#jobsvisits').on('click', 'a.editor_duplicate', function(e) {
e.preventDefault();
editor.create('You must change at least one field in order to copy this row.',
{
"label": "Create new row with this data",
"fn": function () { this.submit(); }
});
var fieldlist = editor.fields();
alert(fieldlist);
var data = editor.fnGetData($(this).parents('tr')[0]);
alert(data);
for (var key in data) {
if ($.inArray(key, fieldlist) >= 0) {
editor.set(key, data[key]);
}
}
});
$('#jobsvisits').dataTable( {
"iCookieDuration": "60*60*24*14", //14 days
"sDom": 'Tfrtip',
"sAjaxSource": "TableEditor/php/table.jobsvisits.php",
"bDeferRender": true,
"bInfo": true,
"sScrollY": "500px",
"sScrollX": "340px",
"bScrollInfinite": true,
"bScrollCollapse": true,
"bPaginate": false,
"bProcessing": true,
"bStateSave": true,
"aoColumns": [
{
"mData": null,
"sClass": "center",
"sDefaultContent": '  '
},
{ "mData": "LeadSource" },
{ "mData": "Visit", "sClass": "center" },
{ "mData": "Pack", "sClass": "center" }
],
"oTableTools": {
"sRowSelect": "single",
"aButtons": [
{ "sExtends": "editor_create", "editor": editor },
{ "sExtends": "editor_edit", "editor": editor },
{ "sExtends": "editor_remove", "editor": editor }
]
}
});
});
var jvisits= new Array({"label" : "a", "value" : "a"});
function get_jvisits(){
jvisits.splice(0,1);
$.ajax({
url: 'TableEditor/php/get_jvisits.php',
async: false,
dataType: 'json',
success: function (json) {
for(var a=0;a
> var data = editor.fnGetData($(this).parents('tr')[0]);
fnGetData isn't a method of Editor - it is a method of DataTables ( http://datatables.net/api#fnGetData ). So I think the browser is right in saying that the function doesn't exist.
Save `$('#jobsvisits').dataTable(..)` to a variable and call that, rather than the Editor instance (assuming it is the `jobsvisits` table you want the data from.
Allan
As you pointed out I just needed to add the line
[code] var dataTableObject=$('#jobsvisits').dataTable()[/code]
before the fnGetData() line
Thankyou for your help