Scroll to position on reload
Scroll to position on reload
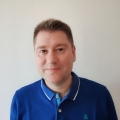
Greetings,
I'm using DataTables client-side to show a table of containers. Each row in turn can be expanded, so that its contents are displayed, in a similar manner of what is done in child rows example. The key difference is that, instead of showing some extra columns in the new expanded row, I create a new child DataTable inside that expanded row, so that it has all the other functionalities of DataTables (data is retrieved from a JSON resource, it has ordering,...). This is working just fine.
Recently, I decided to automatically reload the table every X seconds. For that, I'm using the setInterval, as follows:
1. I'm managing a list of openSubtables to know which are expanded.
2. Once the parent table has been reloaded, their rows are traversed to check whether they should be expanded, and if so, each expanded subtable is recreated once again, asking to the JSON source and so on...
It is something like this:
// This creates a new DataTable intended to be used as a subtable
function subDataTable(vtaskId, tableId) {
return $('table#' + tableId).DataTable({
ajax: {...}
...
});
}
...
// This inserts the subtable into a child row of the containers table
function showSubtable(row, virtualTaskId, visualEffect) {
var subtableId = 'subtable-' + virtualTaskId;
row.child(subtableSkeleton(subtableId), 'noPadding').show();
subDataTable(virtualTaskId, subtableId);
if (visualEffect) {
$('div.subtable', row.child()).slideDown();
}
else {
$('div.subtable', row.child()).show();
}
}
...
// Reloading...
$(function() {
setInterval( function () {
var scrollPos = $('table#containers').scrollTop(); // Gets the scroll
table.ajax.reload(function() {
if (openSubtables.length > 0) {
table.rows().every(function(rowIdx, tableLoop, rowLoop) {
var vtaskId = this.data()['id'];
if (openSubtables.indexOf(vtaskId) > -1) {
showSubtable(this, vtaskId, false); // This recreates the subtable
}
});
}
$('table#containers').scrollTop(scrollPos); // This DOES NOT WORK
}, false ); // user paging is not reset on reload
}, defaultRefreshInterval );
}
As you see, firstly the containers table is reloaded, and once the data is available, every row is traversed to check whether it was expanded. If that's the case, then a new DataTable subtable is created and added as a row.child
Table and opened subtables are correctly reloaded but I'm unable to go back to the previous scrolling position. I have tried different approaches with no luck. I believe that the issue comes from the fact that showSubtables does AJAX calls: Each time it is called, it creates a new DataTable asking for data from a JSON resource. Since those AJAX call are asynchronous, I believe that this prevents the script from reaching its previous position, probably because that position has not been created yet.
Even replacing
$('table#containers').scrollTop(scrollPos); // This DOES NOT WORK
with
setTimeout(function() {
$('table#containers').scrollTop(scrollPos);
}, 2000);
has not helped. There is a small scroll on the first reload, which gets lost after some reloads.
How can I achieve to restore the scroll on this case?
Regards,
Carlos