Field manipulations on the client side aren't passed to the server; they only affect the front end
Field manipulations on the client side aren't passed to the server; they only affect the front end
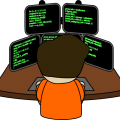
I have a single row editing form; the user enters data and they are subsequently modified using val()
. In my debugger I can see that it works: the data entry fields are definitely modiefied using preSubmit
. But then, surprise: the unmodified values are submitted to the server! I have no idea what to do about this. Please help.
This is my code. Please look at userPhoneEditor
.on( 'preSubmit', function ( e, o, action ) .....
var userPhoneEditor = new $.fn.dataTable.Editor( {
ajax: {
url: 'actions.php?action=tblUserPhone',
data: function ( d ) {
var selected = userTable.row( {selected: true} );
if (selected.any()) {
d.user = selected.data().user.id;
}
}
},
table: "#tblUserPhone",
fields: [ {
label: "Phone Type:",
name: "phone.type",
type: "select",
options: [
{ label: "Work", value: "work" },
{ label: "Mobile", value: "mobile" },
{ label: "Alternate", value: "alternate" }
]
}, {
label: "Country Code:",
name: "phone.countrycode",
id: "phoneCountrycode"
}, {
label: "Area Code:",
name: "phone.areacode",
id: "phoneAreacode"
}, {
label: "Number:",
name: "phone.phone",
id: "phonePhone"
}
]
} );
userPhoneEditor
.on('open', function() {
$.myMask.definitions['b'] = "[0-9 ]";
$.myMask.definitions['c'] = "[0-9-xX ]";
$.myMask.definitions['8'] = "[1-9]";
$("#phoneCountrycode").myMask("+8?bb",{placeholder:"_"});
$("#phoneAreacode").myMask("9?bbbb",{placeholder:"_"});
$("#phonePhone").myMask("89c?cccccccccccc",{placeholder:"_"});
});
userPhoneEditor
.on( 'preSubmit', function ( e, o, action ) {
if ( action !== 'remove' ) {
var countryCode = this.val('phone.countrycode' );
var areaCode = this.val('phone.areacode' );
var phone = this.val('phone.phone' );
//validation with error messages is mostly done on the server and through masking
// only in Italy the first digit of the area code may be zero; other wise the leading zero is cut out
if (countryCode != "+39") {
if (areaCode.substring(0, 1) == "0") {
areaCode = areaCode.substring(1);
}
}
countryCode = countryCode.substring(1);
this.val('phone.countrycode', countryCode);
this.val('phone.areacode', areaCode);
}
} );
userPhoneEditor
.on( 'submitSuccess', function () {
userTable.ajax.reload();
});
var userPhoneTable = $('#tblUserPhone').DataTable( {
dom: "Bfrtip",
ajax: {
url: 'actions.php?action=tblUserPhone',
type: 'POST',
data: function ( d ) {
var selected = userTable.row( {selected: true} );
if (selected.any()) {
d.user = selected.data().user.id;
}
}
},
columns: [
{ data: "phone.type"
, render: function ( data, type, row ) {
if (lang == 'de') {
switch (row.phone.type) {
case "work":
return "Arbeit";
break;
case "mobile":
return "Mobil";
break;
default:
return "Sonstige";
break;
}
} else {
return row.phone.type;
}
}
},
{ data: null,
render: function ( data, type, row ) {
return '+' + row.phone.countrycode + ' ' + row.phone.areacode + ' ' + row.phone.phone;
}
},
{ data: "phone.countrycode" },
{ data: "phone.areacode" },
{ data: "phone.phone" }
],
columnDefs: [
{ targets: [2, 3, 4], visible: false }
],
// sequence: work, mobile, alternate, then ascending by full number
order: [[ 0, 'desc' ], [ 1, 'asc' ]],
select: {
style: 'single'
},
buttons: [
{ extend: "create", editor: userPhoneEditor },
{ extend: "edit", editor: userPhoneEditor },
{ extend: "remove", editor: userPhoneEditor },
"colvis"
]
} );
This question has an accepted answers - jump to answer
Answers
Got it fixed by myself, but I don't like the solution at all. What I am doing right now is to manipulate the object that will be sent to the server directly. What I did before was to manipulate the Editor object using val() which unfortunately had no impact on what was actually sent to the server. Isn't that a bug? And isn't there a nicer solution for this using the API?
Here is my solution for the time being:
You can't use
val()
inpreSubmit
as the values have already been read from the fields when that event is triggered. That event is provided as a hook to let you modify the data that has been read from the form before it is sent to the server.As such, this isn't a but, but rather working as intended.
Allan
ok, if this is intentionally so I am fine with it. Thought I got something wrong ...