How can I use editor.dependent() to get a single value for a field instead of a select?
How can I use editor.dependent() to get a single value for a field instead of a select?
I usually use editor.dependent() to get a value from a list box:
// JS file
...
{
label: "Provincia",
name: "contatti.cont_prov",
type: "select"
},
{
label: "Località",
name: "contatti.cont_loc",
type: "select"
},
{
label: "CAP",
name: "contatti.cont_cap",
type: "select"
},
...
clientiEditor.dependent( 'contatti.cont_prov', 'php/filtro_localita.php' );
clientiEditor.dependent( 'contatti.cont_loc', 'php/filtro_cap.php' );
...
// PHP file: filtro_localita.php
<?php
include( "DataTables.php" );
$comuni = $db
->select( 'comuni', ['com_nome as value','com_nome as label']
,['com_prov' => $_REQUEST['values']['contatti.cont_prov']] )
->fetchAll();
echo json_encode( [
'options' => [
'contatti.cont_loc' => $comuni
]
] );
// PHP file: filtro_cap.php
<?php
include( "DataTables.php" );
$cap = $db
->select( 'comuni', ['com_cap as value','com_cap as label']
,['com_nome' => $_REQUEST['values']['contatti.cont_loc']] )
->fetchAll();
echo json_encode( [
'options' => [
'contatti.cont_cap' => $cap
]
] );
<?php
>
```
But how can I get a single value for the contatti.cont_cap field instead of a list box?
I tried to write:
```
// JS file
...
{
label: "Provincia",
name: "contatti.cont_prov",
type: "select"
},
{
label: "Località",
name: "contatti.cont_loc",
type: "select"
},
{
label: "CAP",
name: "contatti.cont_cap"
?>
},
and I modified the file filtro_cap.php in this way:
```
<?php
include( "DataTables.php" );
$cap = $db
->select( 'comuni', ['com_cap']
,['com_nome' => $_REQUEST['values']['contatti.cont_loc']] )
->fetchAll();
echo json_encode( $cap );
<?php > ``` the php file returns (for example): ``` [{"com_cap":"92100"}] ``` but my field is: contatti.cont_cap... how should i do? ?>Thanks for your help,
Giuseppe
This discussion has been closed.
Answers
should do it I think.
If it doesn't could you add
print_r( $_REQUEST );
and show me the output?Thanks,
Allan
I try to explain myself better...
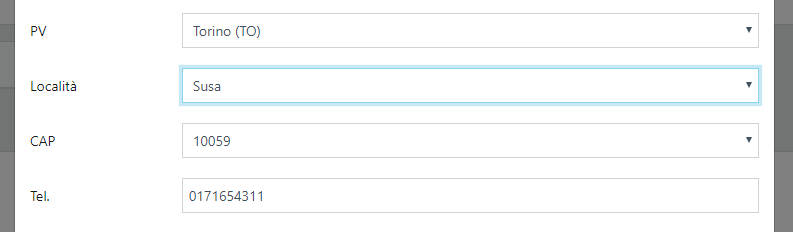
I would like to get the value for the 'contatti.cont_cap' (CAP) field without having to select it from a list box
As you can see from the image, by selecting the 'Localita' field (contatti.cont_loc) I get the value of the 'CAP' field (contatti.cont_cap) because both fields are present in the 'comuni' table (com_nome and com_cap).
I get only one 'CAP' value but I would like it to be editable and it would not look like a list box with only one value ...
Thanks,
Giuseppe
I was able to get the single field value I needed.
I would just like to rewrite the same instructions with the "standard datatables syntax"...
Thanks for the help you want to give me.
Giuseppe