Potential Editor Bug using "Every"
Potential Editor Bug using "Every"
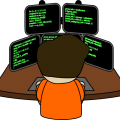
I tried to implement a button that selects table rows depending on the value of a field in the row. I thought using "every" would be a good option and implemented this code:
//custom button to select all rows that are in the accounting interface using "Every"
$.fn.dataTable.ext.buttons.selectAllInterfaceEvery = {
text: selectAllInterfaceLabel + '_Every',
name: "selectedAllInterfaceButton",
action: function ( e, dt, button, config ) {
dt.rows({ search: 'applied' }).every( function ( rowIdx, tableLoop, rowLoop ) {
var data = this.data();
if ( data.contract_has_infoma.include > 0 ) {
dt.row(this).select();
} else {
dt.row(this).deselect();
}
});
}
};
Surprisingly this solution didn't perform at all. It produced dozens of useless ajax calls and it took over 30 seconds to complete. The final result was correct, but the performance was unsatisfactory:
I ended up implementing this code that performs very well:
//custom button to select all rows that are in the accounting interface
$.fn.dataTable.ext.buttons.selectAllInterface = {
text: selectAllInterfaceLabel,
name: "selectedAllInterfaceButton",
action: function ( e, dt, button, config ) {
// var sRows = dt.rows({ search: 'applied' });
var sRows = dt.rows({ search: 'applied' }).data().toArray();
var interfaceArray = [];
var notInterfaceArray = [];
$.each(sRows, function(key, value) {
if ( value.contract_has_infoma.include > 0 ) {
interfaceArray.push("#" + value.DT_RowId);
} else {
notInterfaceArray.push("#" + value.DT_RowId);
}
})
dt.rows(interfaceArray).select();
dt.rows(notInterfaceArray).deselect();
}
};
This wasn't easy either because there is a mistake in the docs.
https://datatables.net/reference/type/row-selector
The "-" should be an "_".
Do you have an explanation why "every" is not performing? Thanks for taking a look!
This question has an accepted answers - jump to answer
Answers
I don't see why the above code would trigger an Ajax call at all since there is no ajax functionality in the above? Or have I missed it?
Good to hear you've got it working now though.
Allan
Exactly, Allan! It does not trigger an Ajax Call. But does the functionality behind "every" trigger such a call? For me this behavior was very surprising and I don't understand it either.
This is the entire javascript code including the two buttons. Can you find anything there that would explain this weird behavior?
Found the reason: on every deselect I do an ajax reload of the data table. And that happens many times when using the above logic with "Every". It only happens once when using the array based solution that I implemented!
Looks like you are building an array in the
each
solution then after the loop you are doing one select and one deselect. Where in theevery
loop you are individually selecting or deselecting rows. If you were to build an array like theeach
solution then you would have only one ajax call.Kevin
Amazing! same thought at the same time, Kevin!
This is where I do the ajax call after every deselect:
No bug! But the array based solution is certainly the best in this case because I can't get rid of the ajax reload after deselecting a row.