MySQL's SUM() function in global validator doesn't work
MySQL's SUM() function in global validator doesn't work

I'm trying to add all the values in a percentage column in a global validator to check user input against but it kept on returning the string in the return statement when ran, even though the total sum doesn't exceed 100. For that, I'm using MySQL's SUM() function as follows:
->validator(function($editor, $action, $data){
if ($action === Editor::ACTION_CREATE || Editor::ACTION_EDIT){
foreach ($data['data'] as $pkey => $values){
$sum = $editor->$db()->query('select')->get('SUM(percentage)')->table('assessment_items')
->where('assessment_items.subject_details', $_SESSION['details'])
->exec()
->fetch();
if ($sum > 100){
return 'The total value should not exceed 100%';
}
}
});
Any pointer is greatly appreciated.
This question has accepted answers - jump to:
This discussion has been closed.
Answers
What was
$sum
? If you add:it will cause a JSON error, but you'll be able to see what $sum is.
I suspect you might need something like:
and change
->get('SUM(percentage)')
to be->get('SUM(percentage) as total')
. The reason being -$sum
is the row (i.e. an array), not just a single value. Theas
alias is just to make it easier to read.Allan
I should also point out that the validator runs before the database values are written. So the above doesn't actually take into account the values for what the user is sumbitting! Only what are already in the database. You'd need to merge the newly submitted values with those from the database. A
sum
in PHP is probably the easiest way of doing that - i.e. select the rows and their id's, then check the submitted data to see if a new value was submitted, if so add it to the sum, if not use the db value.Allan
Thank you Allan for guiding me in the right direction.
I have altered my validator as per your suggestions as follows:
I now have this unexpected error when creating/editing a record:
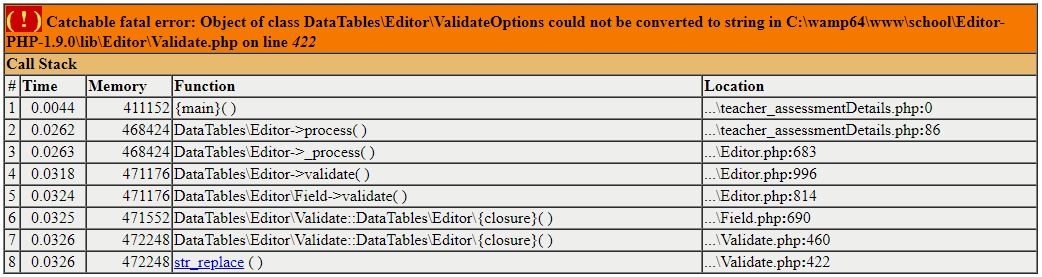
I really appreciate any help in this.
That line is in a numeric validator. Do you have any numeric validation functions in your code? If so, could you show them to me please?
Allan
Thank you Allan!
The numeric validator that seems to be causing this is:
Let me know if I've gone wrong somewhere. For now, I've changed the above to the following custom validator (which worked):
Here is the working code for the global validator taking into account your feedback on merging newly submitted value with the the existing total in the database:
Thank you so much Allan!