Inline Editor, Dropdown list, bind data to list
Inline Editor, Dropdown list, bind data to list
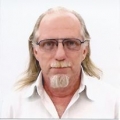
I have went through discussion and doc but can not seem to find a solution to my problem. I have a column that is tied to a second table. I have built the data from the table to be displayed in the dropdown list but I can not seem to connect the collection to the list. When looking at the json data I can see the collection called 'Location'. Basically I get the drop arrow but when click it there is nothing in the list.
I would appreciate any help I can get.
This the controller:
using (var dbe = new DataTables.Database(Globals.DbType, Globals.DbConnection))
{
int tripmasterID = Convert.ToInt32(Session["tripmasterID"]);
var response = new Editor(dbe, "MasterStops", "ID")
.Model<ViewModels.JoinModelMastStops>("MasterStops")
.Model<ViewModels.JoinModelSMSStops>("sms_stops")
.Field(new Field("MasterStops.USPSTrip"))
.Field(new Field("MasterStops.SMS_Stage"))
.Field(new Field("sms_stops.LOCATIONNM", "Location")
.Options(new Options()
.Table("sms_stops")
.Value("ID")
.Label("LOCATIONNM")
)
)
.Field(new Field("sms_stops.STOPTYPE"))
.Field(new Field("sms_stops.Nass_Code"))
.Field(new Field("MasterStops.SchArvTime"))
.Field(new Field("MasterStops.SchDepTime"))
.Field(new Field("MasterStops.DayNumber"))
.Field(new Field("MasterStops.StopNumber"))
.LeftJoin("sms_stops", "MasterStops.ID", "=", "sms_stops.ID")
.Where("MasterStops.TripMastID", tripmasterID)
.Process(request)
.Data();
return Json(response, JsonRequestBehavior.AllowGet);
}
The Java script:
document.getElementById("Loading").style.display = "none";
$(document).ready(function () {
$(function () {
$(".checkinout").mask("99:99");
$(".time").mask("99:99", { placeholder: "0" });
//$(".miles").mask("9999.99", { placeholder: "_" });
$(".hours").mask("999.99", { placeholder: "0" });
$(".initials").mask("aaa");
});
$(".dtp").datepicker();
var editor = new $.fn.dataTable.Editor({
ajax: '@Session["RootFolder"]' + '/TripMasters/Table',
table: '#schedtable',
fields:
[
{ label: 'POTRIP', name: 'MasterStops.USPSTrip' },
{ label: 'Stage', name: 'MasterStops.SMS_Stage' },
{ label: 'Stop Name', name: 'MasterStops.SMS_StopsID', type: "select" },
{ label: 'StopType', name: 'sms_stops.STOPTYPE' },
{ label: 'Nass', name: 'MasterStops.Nass_Code' },
{ label: 'Arrive', name: 'MasterStops.SchArvTime' },
{ label: 'Depart', name: 'MasterStops.SchDepTime' },
{ label: 'Day #',
name: 'MasterStops.DayNumber',
type: "select",
options:
[
{ Label: '1', value: '1' },
{ Label: '2', value: '2' },
{ Label: '3', value: '3' }
]
},
{ label: 'Stop Order', name: 'MasterStops.StopNumber' }
]
})
$('#schedtable').on('click', 'tbody td.editable', function (e) {
editor.inline(this, {
onBlur: 'submit'
});
});
$('#schedtable').DataTable({
dom: 'Bfrtip',
ajax: '@Session["RootFolder"]' + '/TripMasters/Table',
model: 'JoinMastStops',
"scrollY": "300px",
"scrollCollapse": true,
"paging": false,
"bFilter": false,
"paging": false,
"bFilter": false,
"order": [[8, 'asc']],
columns:
[
{ data: 'MasterStops.USPSTrip', className: 'editable' },
{ data: 'MasterStops.SMS_Stage', className: 'editable' },
{ data: 'sms_stops.LOCATIONNM', className: 'editable', editField: "MasterStops.SMS_StopsID" },
{ data: 'sms_stops.STOPTYPE', className: 'editable' },
{ data: 'MasterStops.Nass_Code', className: 'editable' },
{ data: 'MasterStops.SchArvTime', className: 'editable' },
{ data: 'MasterStops.SchDepTime', className: 'editable' },
{ data: 'MasterStops.DayNumber', className: 'editable' },
{ data: 'MasterStops.StopNumber', className: 'editable' }
],
columnDefs:
[
{ "targets": [0], "orderable": false },
{ "targets": [1], "orderable": false },
{ "targets": [2], "orderable": false },
{ "targets": [3], "orderable": false },
{ "targets": [4], "orderable": false },
{ "targets": [5], "orderable": false },
{ "targets": [6], "orderable": false },
{ "targets": [7], "orderable": false },
{ "targets": [8], "orderable": false },
],
select: true,
//select: {
// style: 'os',
// collection: 'Location'
//},
buttons:
[
//{ extend: "create", editor: editor },
//{ extend: "edit", editor: editor },
//{ extend: "remove", editor: editor }
]
})
});
This question has an accepted answers - jump to answer
Answers
I might not quite be getting something, but in your Editor JS configuration you have two fields which are
select
:On the server-side you have a single field which is using the
Options
class:I don't see that field in the client-side Editor. So I'm a little confused as to which field those options should be appearing in?
Thanks,
Allan
In my original post I failed to post the ViewModel for the two tables.
MasterStops is the main table. The field SMS_StopsID joins the sms_stops table to get ‘LOCATIONNM’. The select field needs to show the ‘LOCATIONNM’ with the value being the sms_stops.ID and stored in MasterStops.sms_stopsID. Hope this helps explain better. The issue is that the dropdown list contains no values.
}
also here is the new javascript:
Thank you for the additional information - try this:
That will get the value for the field from
MasterStops.SMS_StopsID
and then usesms_stops
entries for the dropdown list.Allan
Thank you so much. I spent 3 days trying to solve the problem. Have a blessed day.