Invalid JSON Data with Newtonsoft JSON Serializer
Invalid JSON Data with Newtonsoft JSON Serializer

Hi, I'm making a web app using ASP.NET Core and Entity Framework Core.
And I have a table called Items. I pass the list of items to DataTables using Newtonsoft JSON Serializer.
I don't know why I got this error every time I have a lot of Item data, but this doesn't happen in other lists / DataTables (yet).
DataTables warning: table id=ItemTable - Invalid JSON response. For more information about this error, please see http://datatables.net/tn/1
Currently, I have 21 rows of Item data. And this actually happened several times.
1. I use this code and my data is at 15 rows, got that error.
public string GetAll()
{
var model = _itemRepository.GetAll();
var settings = new JsonSerializerSettings
{
ReferenceLoopHandling = ReferenceLoopHandling.Ignore
};
var serialized = JsonConvert.SerializeObject(model, Formatting.Indented, settings);
return serialized;
}
2. I've changed the code to this, I can store up to 17-21 rows and after row 21 I get that error again.
public string GetAll()
{
var model = _itemRepository.GetAll();
var settings = new JsonSerializerSettings
{
ReferenceLoopHandling = ReferenceLoopHandling.Ignore
};
var serialized = JsonConvert.SerializeObject(model, settings);
return serialized;
}
This is my DataTable inside my Item List view
var table = $("#ItemTable").DataTable({
"processing": true,
"ajax": {
"url": "Item/GetAll",
"dataSrc": ""
},
"columnDefs": [
{
"targets": 0,
"title": "Id",
"data": "Id",
"visible": false
},
{
"targets": 1,
"title": "Brand",
"data": "Brand.Name"
},
{
"targets": 2,
"title": "Name",
"data": "Name"
},
{
"targets": 3,
"title": "Price",
"data": "Price",
"render": $.fn.dataTable.render.number( ',', '.', 2, 'Rp ' ),
"searchable": false
},
{
"targets": 4,
"title": "UoM",
"data": "UoM.Name"
},
{
"targets": 5,
"title": "Category",
"data": "Category.Name"
},
{
"targets": 6,
"title": "Stock On Hand",
"data": "StockOnHand",
"render": $.fn.dataTable.render.number( ',' ),
"searchable": false
},
{
"targets": 7,
"title": "On Reserved",
"data": "OnReserved",
"render": $.fn.dataTable.render.number( ',' ),
"searchable": false
},
{
"targets": 8,
"title": "Actions",
"data": null,
"searchable": false,
"orderable": false,
"render": function (data, type, row) {
if (data.ActiveStatus == "Active") {
return "<div class=\"btn-group\">" +
"<button type=\"button\" class=\"btn btn-primary dropdown-toggle\" data-toggle=\"dropdown\" aria-haspopup=\"true\" aria-expanded=\"false\">" +
"Actions" +
"</button>" +
"<div class=\"dropdown-menu\">" +
"<a id=\"Detail\" class=\"dropdown-item\" href=\"#\"><i class=\"fas fa-stream\"></i> Details</a>" +
"<a id=\"Edit\" class=\"dropdown-item\" href=\"#\"><i class=\"fas fa-pen\"></i> Edit</a>" +
"<div class=\"dropdown-divider\"></div>" +
"<a id=\"SetInactive\" class=\"dropdown-item\" href=\"#\"><i class=\"far fa-square\"></i> Inactive</a>" +
"<div class=\"dropdown-divider\"></div>" +
"<a id=\"Delete\" class=\"dropdown-item\" href=\"#\"><i class=\"fas fa-trash\"></i> Delete</a>" +
"</div>" +
"</div>";
}
else if (data.ActiveStatus == "Inactive") {
return "<div class=\"btn-group\">" +
"<button type=\"button\" class=\"btn btn-primary dropdown-toggle\" data-toggle=\"dropdown\" aria-haspopup=\"true\" aria-expanded=\"false\">" +
"Actions" +
"</button>" +
"<div class=\"dropdown-menu\">" +
"<a id=\"Detail\" class=\"dropdown-item\" href=\"#\"><i class=\"fas fa-stream\"></i> Details</a>" +
"<a id=\"Edit\" class=\"dropdown-item\" href=\"#\"><i class=\"fas fa-pen\"></i> Edit</a>" +
"<div class=\"dropdown-divider\"></div>" +
"<a id=\"SetActive\" class=\"dropdown-item\" href=\"#\"><i class=\"far fa-check-square\"></i> Active</a>" +
"<div class=\"dropdown-divider\"></div>" +
"<a id=\"Delete\" class=\"dropdown-item\" href=\"#\"><i class=\"fas fa-trash\"></i> Delete</a>" +
"</div>" +
"</div>";
}
}
}
],
"order": [[2, "asc"]],
"createdRow": function (row, data, dataIndex) {
if (data.ActiveStatus == "Inactive") {
$(row).addClass("table-warning");
}
}
});
This happens only on Item. Loading data takes a little bit too long. When I check the output of the GetAll method, it returns JSON like string, and it is a very long and I think it never ends, just keep scrolling.
Please help. If you need my code, I could send them.
Thank you, kind regards
Michael Reno
Answers
That error would occur if the JSON doesn't match what's expected. Can you post the response here, please, then we can compare with what DataTables is expecting,
Colin
Hi, sure, but as I told you, it keeps on going, with no end
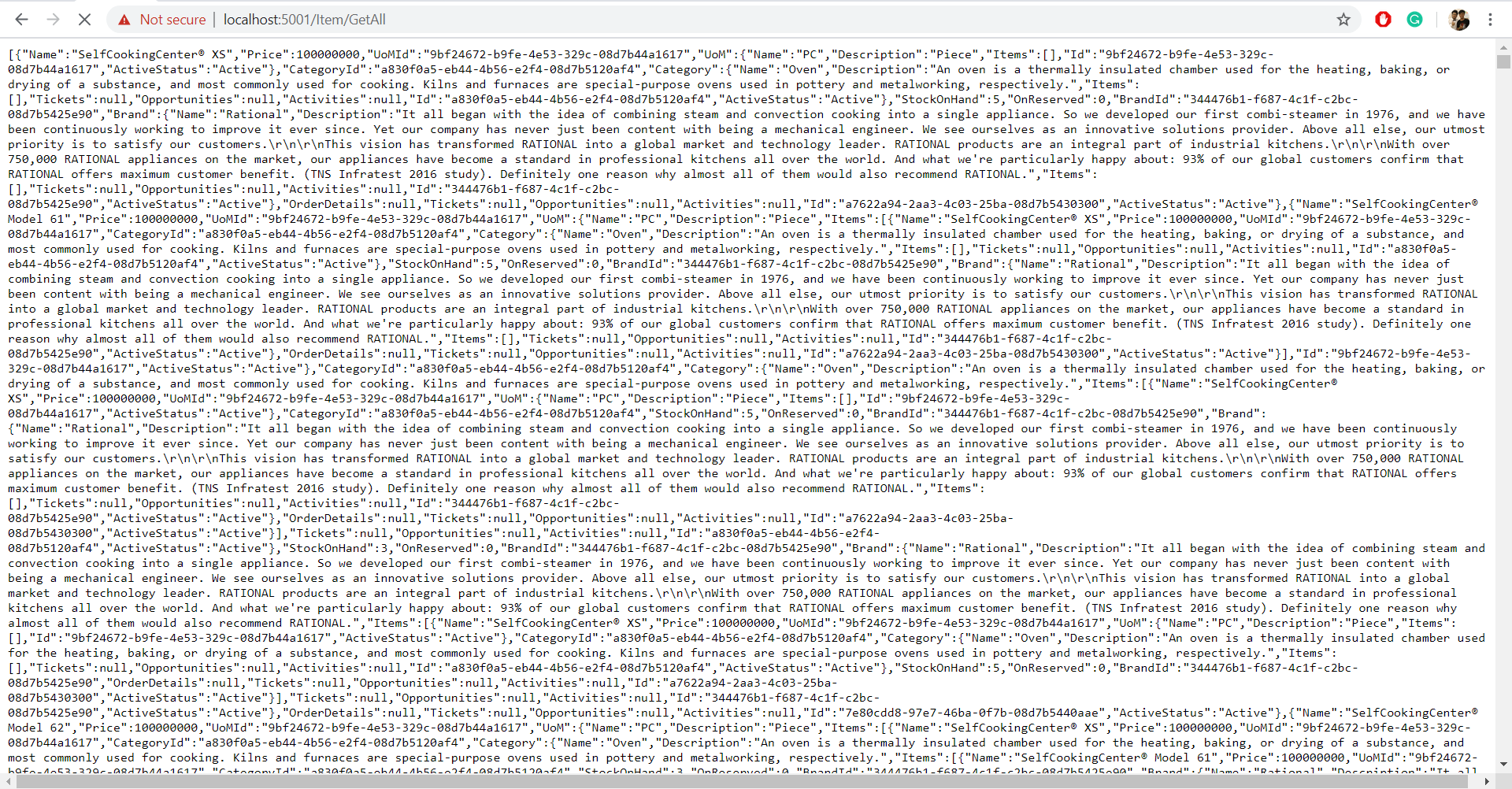
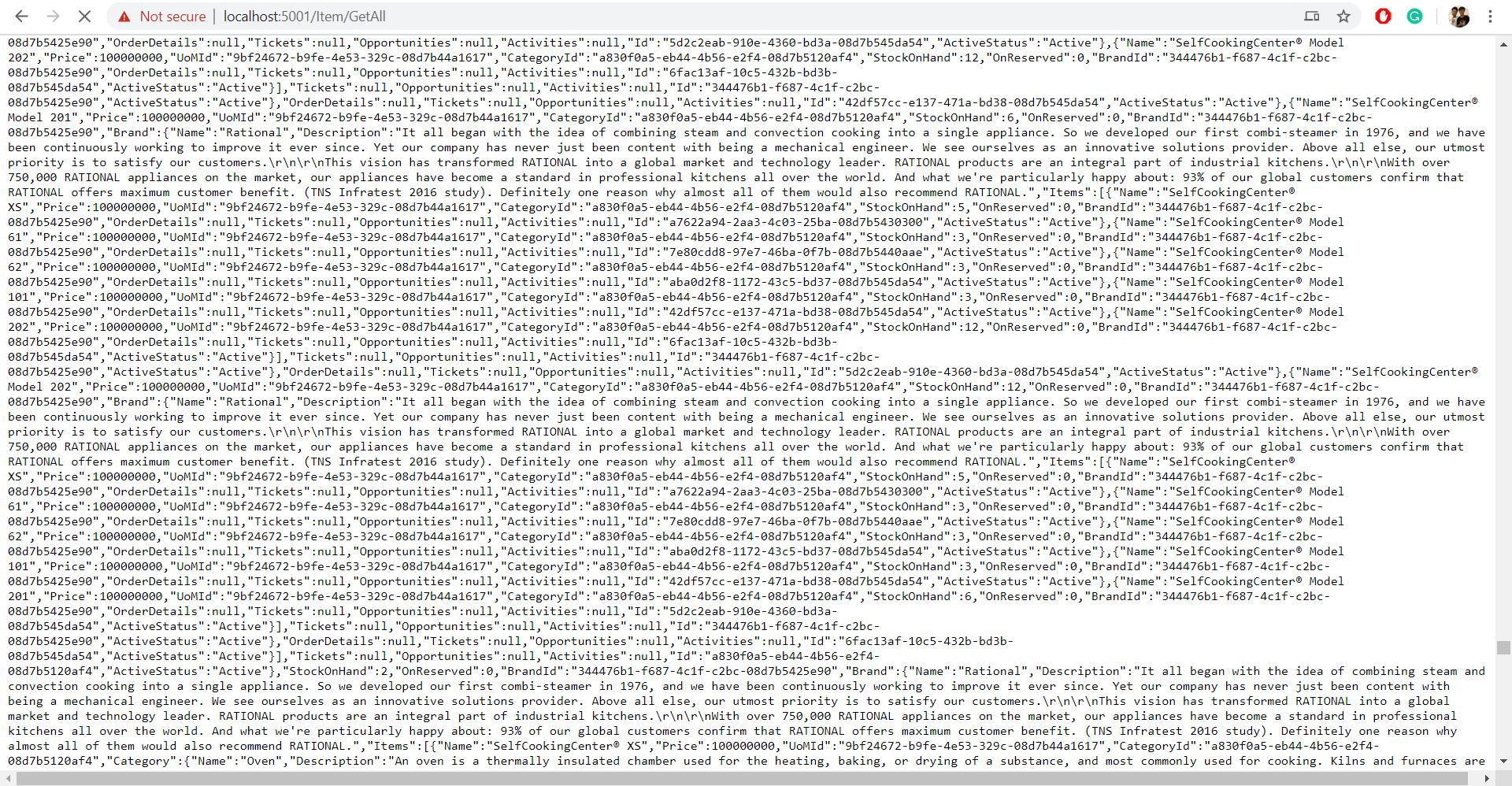
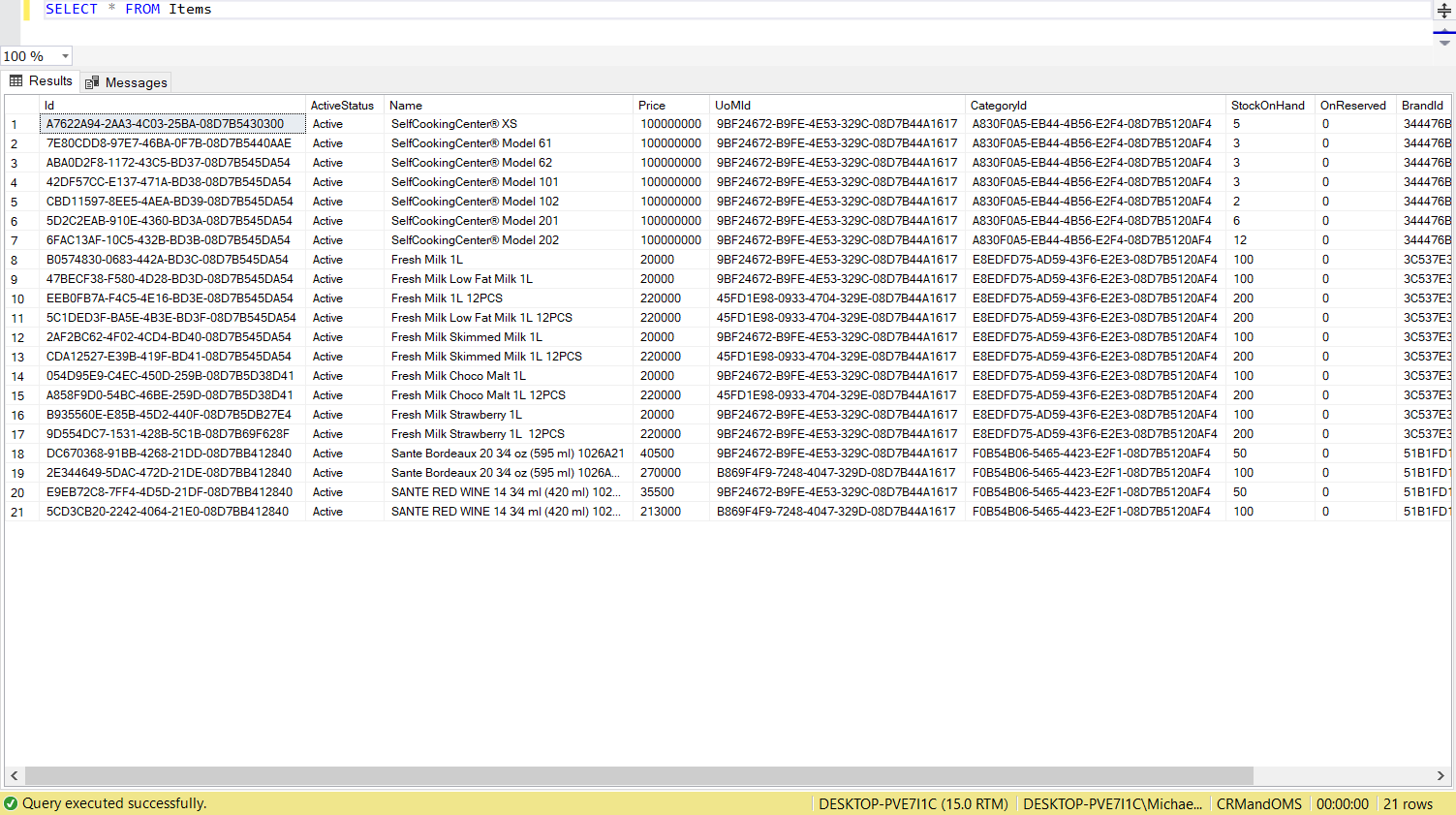
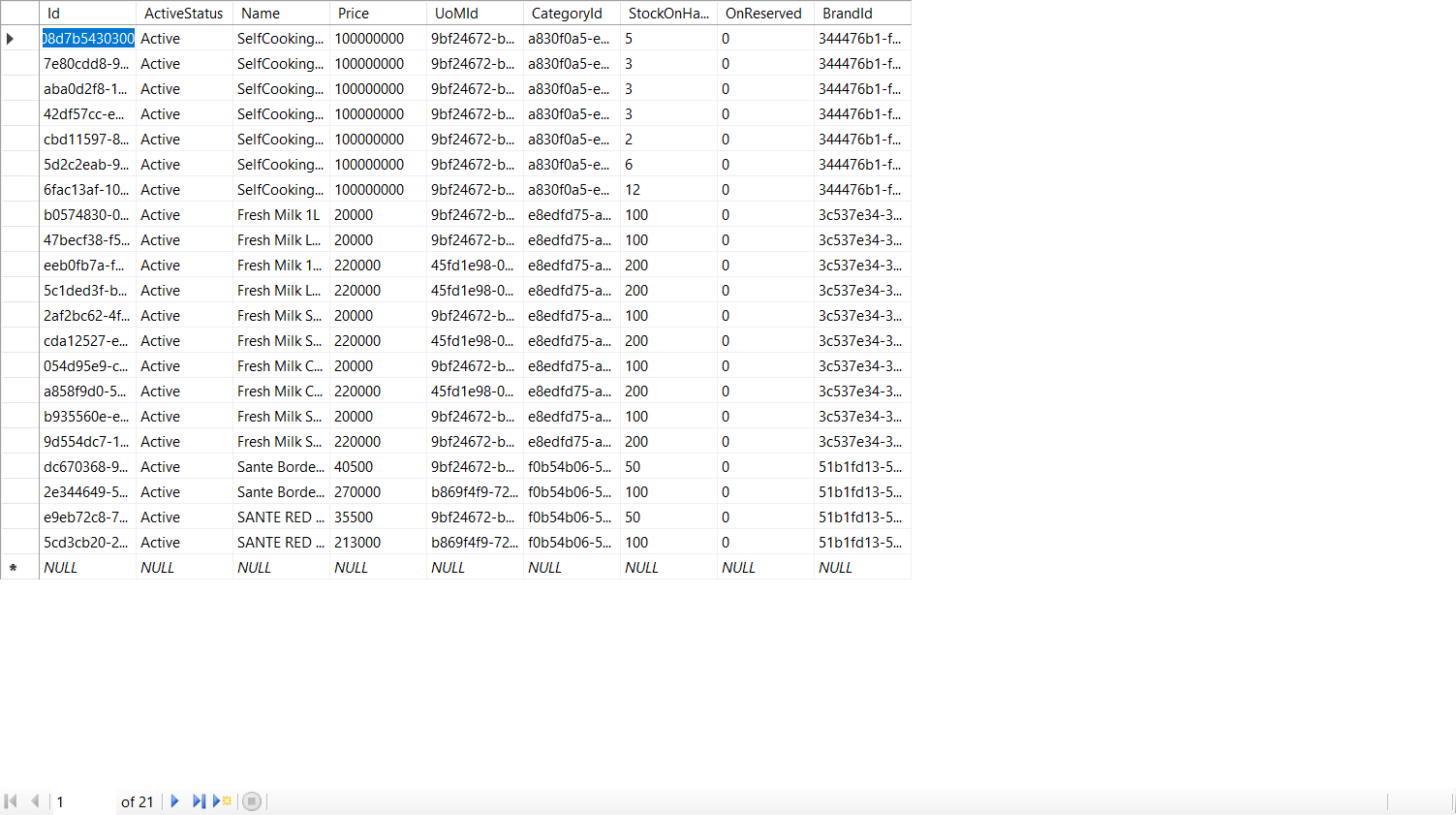
This one I just arrived at the bottom of the page, and it keeps on loading more and more and loop and loop.
And I only have 21 rows of Items data
Are you saying there is more than 21 rows of data?
Seems like there is a lot of data there. There is a Description field which you don't seem to be using in your table.
You can use https://jsonlint.com/ to validate the JSON is a valid string.
This doesn't sound like a Datatables issue but more an issue with how your script is fetching the data. I would start with debugging that to make sure its fetching the expected data then use https://jsonlint.com/ to validate if you still get the
Invalid JSON response
error. The link in the error has some troubleshooting steps.Kevin
In my database there are 21 rows of data
But when I load it, it keeps on loading more than the data inside the database. So it loops. Endlessly.
DataTables just loads the information given in the Ajax response. So as Kevin said, It looks like the issue is that the server is giving the wrong response.
If that doesn't help, we're happy to take a look, but as per the forum rules, please link to a test case - a test case that replicates the issue will ensure you'll get a quick and accurate response. Information on how to create a test case (if you aren't able to link to the page you are working on) is available here.
Cheers,
Colin
Can I just give you the link to my code?
If it's running, we really need to see it fail.
Colin
It's failing. I've tried it on many of my computers.
Here you go : https://drive.google.com/open?id=12u2z5BokyIXU1WNl26vg1xEKcotPKHAm
Colin is asking for a link to a running test case.
Sorry I don't have that link. I have the source code.
If the server script
Item/GetAll
is responding with the incorrect data then that is what needs to be debugged by adding debug statements or invoking the debugger in your environment.Kevin