Everytime re-open the form, editor is on change fired.
Everytime re-open the form, editor is on change fired.
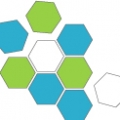
Hi all,
I wish to modify my instance of Editor in the JS file, such that a field of type select ('event.event_1') can change its options to values created from another database, based on what value I selected in the field ('event_operator').
I found this discussion link: https://datatables.net/forums/discussion/23918, but there are problems with the re-opening of the editor.
Until then, it works perfectly to the point of selection and store to the database. But at re-open the editor, the value in the field ('event.event_1') was reseted. However, the event.operator value is OK.
event.js
$(document).ready(function() {
editor = new $.fn.dataTable.Editor( {
ajax: "controllers/staff_event.php",
table: "#example",
fields: [
{
type: "select",
label: "Number of events",
name: "event.num_of_events",
placeholder: 'Please select',
options: [
"1",
"2",
"3",
"4"
]
}, {
type: "select",
label: "Operator:",
name: "event.operator",
placeholder: "Please select operator"
}, {
type: "select",
label: "Event 1:",
name: "event.event_1",
placeholder: "Please select event 1"
},
......
columns: [
{ data: "event.event_1" },
{ data: "event.event_2" },
{ data: "event.event_3" },
......
// AJAX Call for content of events
$(editor.field('event.operator').node()).on('change', function (e, d) {
var grpID = editor.field('event.operator').val();
console.log("on change fired");
if (!d) {
console.log("user change fired");
editor.submit();
}
$.ajax({
url: "controllers/staff_event_operator.php",
data: {
grpID: grpID
},
dataType: 'JSON',
success: function (json) {
editor.field('event.event_1').update(json);
}
});
});
File read out the stuff for the select field ('event.event_1'):
staff_event_operator.php
<?php
$tempOperatorID = $_GET["grpID"];
require_once '../inc/db.php';
$event_choice = "SELECT veranstaltung.id, veranstaltung.db_vdate, veranstaltung.db_vtime, veranstaltung.db_title, veranstaltung.db_operator
FROM veranstaltung
WHERE veranstaltung.db_operator = $tempOperatorID ";
$mysqli = new mysqli($db_host, $db_user, $db_pass, $db_name);
$mysqli->set_charset('utf8');
$data = array();
if ( !isset($POST['action']) ) {
if ($event_result = $mysqli->query($event_choice)) {
while ($row = $event_result->fetch_assoc()) {
$data[] = array(
"value" => $row['id'].' - '.$row['db_vdate'].' - '.$row['db_vtime'].' - '.$row['db_title'],
"label" => $row['id'].' - '.$row['db_vdate'].' - '.$row['db_vtime'].' - '.$row['db_title']
); }
// $data[] = $data;
$event_result->free();
}
}
echo json_encode($data);
I checked this with the console logger. Everytime re-open the form, editor is on change fired. Where is my mistake?
Thanks for helping
Mac
This question has an accepted answers - jump to answer
Answers
THe
node()
will change when the form is opened, so thatchange
event is being correctly triggered.It sounds like you need to use
dependent()
onevent_operator
to initiate the Ajax request. There's an example ofdependent()
here,Colin
Hi Colin,
thanks for the reply and the tip. It works now with the dependent function. But I have a problem with this 3 strips next to the selection field, because with the callback I can't return the array[]. I need to update it with the function editor.field. Or is there another solution?
event.js
staff_event_operator.php
```php
<?php
$tempOperatorID = $_GET["grpOperator"];
// DataTables PHP library
require_once '../inc/db.php';
// Datum umwandeln
$event_choice = "SELECT veranstaltung.id AS ver_id, veranstaltung.db_title, veranstaltung.db_vdate, veranstaltung.db_vtime, veranstaltung.db_operator, veranstaltung.db_location, veranstaltung.db_vort, locations.id, locations.location, vortragsort.id, vortragsort.vort
FROM veranstaltung
LEFT JOIN locations ON locations.id = veranstaltung.db_location
LEFT JOIN vortragsort ON vortragsort.id = veranstaltung.db_vort
WHERE veranstaltung.db_operator = $tempOperatorID
GROUP BY veranstaltung.db_vdate
ORDER BY veranstaltung.db_operator, veranstaltung.db_vdate";
$mysqli = new mysqli($db_host, $db_user, $db_pass, $db_name);
$mysqli->set_charset('utf8');
$data = array();
if ( !isset($POST['action']) ) {
echo json_encode($data);
<?php > ``` ?>Thanks again for the great support....
Mac
Hi Mac,
Two options here:
callback({});
since you've already handled the updates you need. That empty object let's Editor know you are finished with the dependent call.dependent()
can process, so you can just docallback(json)
. For that, in your PHP you could do:Regards,
Allan