Collapsible/Expandable Rows
Collapsible/Expandable Rows

Hello, my DataTable is almost exactly where I need it to be. I was able to finally get the row grouping to work, but now I want to make the parent row be able to collapse/expand but cannot figure out how. I looked at this example from this thread, but could not quite understand it as the data in that example was hard coded in the HTML. Here is my code.
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://cdn.datatables.net/1.10.15/js/jquery.dataTables.js"></script>
<script src="https://momentjs.com/downloads/moment.min.js"></script>
<script src="https://cdn.datatables.net/buttons/1.6.2/js/dataTables.buttons.min.js"></script>
<script src="https://cdn.datatables.net/buttons/1.6.2/js/buttons.flash.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jszip/3.1.3/jszip.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.53/pdfmake.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.53/vfs_fonts.js"></script>
<script src="https://cdn.datatables.net/buttons/1.6.2/js/buttons.html5.min.js"></script>
<script src="https://cdn.datatables.net/buttons/1.6.2/js/buttons.print.min.js"></script>
<script src="https://cdn.datatables.net/rowgroup/1.1.2/js/dataTables.rowGroup.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/1.10.15/css/jquery.dataTables.min.css" />
<div class="header">
<h1><strong>Deliverables</strong></h1>
</div>
<div class ="container">
<table id="myTable" class="display" cellspacing="0" width="100%">
<thead>
<tr>
<th>Program</th>
<th>Deliverable</th>
<th>To</th>
<th>Date</th>
<th>Approved</th>
<th>Notes</th>
</tr>
</thead>
<tfoot>
<tr>
<th>Program</th>
<th>Deliverable</th>
<th>To</th>
<th>Date</th>
<th>Approved</th>
<th>Notes</th>
</tr>
</tfoot>
</table>
</div>
<style> //These are just CSS styling I chose to use.
div.container {
min-width: 980px;
margin: 0 auto;
}
.header {
padding: 10px;
text-align: center;
}
body {
font: 90%/1.45em "Helvetica Neue", HelveticaNeue, Verdana, Arial, Helvetica, sans-serif;
margin: 0;
padding: 0;
color: #333;
background-color: #fff;
}
</style>
<script>
function loadData() { //Initializing the AJAX Request function to load in the external list data from different subsites
//create an array of urls to run through the ajax request instead of having to do multiple AJAX Requests
var urls = ["url1","url2","url3"];
for (i=0; i < urls.length; i++) { //for loop to run through the AJAX until all URLs have been reached
$.ajax({
url: urls[i],
'headers': { 'Accept': 'application/json;odata=nometadata' },
success: function (data) { // success function which will then execute "GETTING" the data to post it to a object array (data.value)
data = data;
var table = $('#myTable').DataTable();
table.rows.add( data.value ).draw();
}
});
} // missing bracket
}
$(document).ready(function() {
$('#myTable').DataTable( {
"columns": [
{ "data": "Program", visible: false },
{ "data": "Deliverable" },
{ "data": "To" },
{ "data": "Date" },
{ "data": "Approved" },
{ "data": "Notes" }
],
dom: 'Bfrtip',
buttons: [
'copy','csv','excel','pdf','print'
],
rowGroup: {
dataSrc: 'Program'
}
} );
loadData();
} );
</script>
So I have the rowgroup based off the program attribute, and the rest of the information is under that. Is it possible to have that row collapse/expand all the child rows under it? I will add a picture of what my table looks like
Replies
The source fo the data doesn't matter. Basically it uses
var collapsedGroups = {};
to keep track of the collapsed groups and updates it in therowGroups.startRender
function. The click event is used to toggle the group's object incollapsedGroups
which is then used inrowGroups.startRender
hide, usingdisplay: none;
CSS, to hide the collapsed groups. To get a better understanding use console.log statements or the browser's debugger to see how thecollapsedGroups
variable is used.If you have specific questions let us know but for the most part you should be able to copy and paste the code into your script. Some changes are likely. The best option is to start a test case so we can help. You can start with one of the test cases we built in one of your other threads.
Kevin
@kthorngren Ill add the test case in 1 sec, I tried it and no datatable appears.
http://live.datatables.net/tijehexe/1/edit urls are just there as placeholders because like I have previously said, the sharepoint urls I am pulling from do not work outside of the site.
You need to use simulated data. I built an example for you previously to show how to do this:
https://jsfiddle.net/tx8ahnLz/
If you don't load any data then there is nothing to look at in the test case. Also you have syntax errors to fix so the test case runs. Use the browser's console to see the errors.
Kevin
@kthorngren I am trying to make a nice test case for you, but for some reason I can't get this data to post to my datatable. When I check the console --> network --> XHR, the GET request are there. https://jsfiddle.net/3fgq46b0/
Nevermind, I just needed to change data.value to data
You aren't understanding what I'm saying. Take out all of the Ajax code and use simulated data. For your question we don't care about the Ajax call. We just need some data in the table to work with. You can get a sample from the network inspector or you can just make something up.
Kevin
I do understand what you are saying, whats the difference if I use an ajax call or not? It is still doing the same thing with the data??
The difference is the ajax call fails and there is no data so there is noting we can do with the test case. Using simulated data will populate the table with some data we can use.
Kevin
I just created a test case where the AJAX doesn't fail and you can see in the link I provided https://jsfiddle.net/z9qfne4v/1/
I just changed the column names to go with the data that the request is pulling.
Ok, so I know you are anti - ajax, but this works, the userId is the adult row, and everything else is in the child row. https://jsfiddle.net/z9qfne4v/2/ I copied the code from http://live.datatables.net/cecosoru/1/edit but it creates the row and the row that I want to collapse does have the number of items in it, but neither collapses or expands
I'm not anti ajax. Up until now none of your test cases successfully loaded ajax data. I was trying to show an alternative. I'm all for creating an ajax loaded test case if it works
There is one issue with why the test case doesn't collapse the group. The
tr.group-start
selector for the click event is incorrect from the example. Inspecting the page it looks like it is changed todtrg-start
. I updated your example and it works now:https://jsfiddle.net/twu6d1zb/
Kevin
Kevin,
Thank you. I have no idea how it works on the test case then when I bring it back to notepad++ to switch it to work with my sharepoint site. No datatable even populates...
When the page doesn't load properly then you likely are getting a Javascript error. Take a look at the browser's console.
Kevin
I changed everything back to what I need for my DataTable, and now the ajax doesn't work??? I don't understand haha this is getting on my nerves. Here is my JSFiddle https://jsfiddle.net/raw43p97/
These are the only errors I get. But the second error I always get even when it works properly
Uncaught SyntaxError: Unexpected identifier
Uncaught TypeError: Cannot read property 'Url' of undefined
at Object.success (suiteux.shell.crossdomainproxyiframe.c8a9d4c9cf498eb75fb5.js:114)
at u (suiteux.shell.crossdomainproxyiframe.c8a9d4c9cf498eb75fb5.js:93)
at Object.fireWith [as resolveWith] (suiteux.shell.crossdomainproxyiframe.c8a9d4c9cf498eb75fb5.js:93)
at C (suiteux.shell.crossdomainproxyiframe.c8a9d4c9cf498eb75fb5.js:93)
at XMLHttpRequest.<anonymous> (suiteux.shell.crossdomainproxyiframe.c8a9d4c9cf498eb75fb5.js:93)
startRender: function(rows,group){
it says this is the unexpected identifier
That will cause script problems. Does it give the line number? Look there to see what the syntax error is.
Kevin
This makes no damn sense.
Uncaught SyntaxError: Unexpected token '<' it says at line 651 which I dont even have 120 lines of code
I might actually be the biggest idiot... I forgot to add </script>....
@kthorngren So this is what I have right now, is it possible to rowgroup more than one row? I also want/need to sort through the Deliverables under the program, and make two more clickable rows as Monthly Status Report & Meeting minutes.
@kthorngren and I tried the orderFixed option, and it screws up my DataTable making it look like this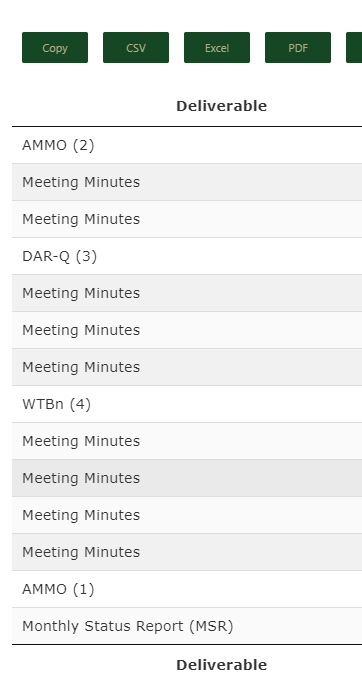
Sorry to spam this post, but to the most recent comment I was able to fix the orderFixed bc I didn't account for a column showing in the array index I had 1 instead of 0 so now it works like a charm