Javascript data from a previous ajax request
Javascript data from a previous ajax request
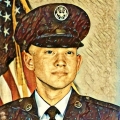
This is admittedly a JS question rather than a DataTables proper question, but I'm turning to the most intelligent, handsome, and friendliest bunch of programmers I know before heading to the callous lion's den of stackoverflow :-)
I have a DataTable that I want to populate with data from a JS object that is the result of a previous jQuery ajax call.
Using this basic example:
https://datatables.net/examples/data_sources/js_array.html
I'm setting it up this way:
var dataSet;
$.ajax({
type: "GET",
url: "datafile.php",
dataType: "json",
success: function (data) {
dataSet = data;
}
});
$(document).ready(function () {
$('#example').DataTable({
data: dataSet,
columns: [
{title: "Name"},
{title: "Position"},
{title: "Office"},
{title: "Extn."},
{title: "Start date"},
{title: "Salary"}
]
});
});
However dataSet
is undefined when the DataTable is created.
I'm certain it's an asynchronous timing issue because I'm initializing the DataTable before the variable "dataSet" has been returned, but any suggestions on how I should structure this?
=== Just extra explanation ==
My actual case involves returning a JS object with multiple data sets, each of which will be used in a table of its own, so that's why I'm trying this route instead of using the ajax request from DataTables itself.
Basically:
var dataSet;
$.ajax({
type: "GET",
url: "datafile.php",
dataType: "json",
success: function (data) {
dataSet = data;
// Looks like dataSet.staff, dataSet.locations, dataSet.titles, etc.
}
});
$(document).ready(function () {
$('#staffTable').DataTable({
data: dataSet.staff,
columns: [
...
]
});
$('#locationsTable').DataTable({
data: dataSet.locations,
columns: [
...
]
});
$('#titlesTable').DataTable({
data: dataSet.titles,
columns: [
...
]
});
});
This question has an accepted answers - jump to answer
Answers
Move your Datatables initialization into the
success
function, like this:Kevin
Thanks, Kevin.
Why would or why wouldn't it go inside this statement?
And how would I include an editor on one of the tables?
Because of the asynchronous processing of Ajax you need to load the Datatable data using the
success
function. You could do something like this if you want to init Datatables first then load the data:Like you normally would. The Editor initialization is independent of Datatables, except where you add the buttons using the
editor
veriable.Kevin
Thanks, Kevin (@kthorngren),
I'm self-taught, so I've managed to make it so far without needing to learn much about asynchronous processing, but as I'm trying to do things that would improve my site I'm being pushed into areas I'm less familiar with.
Along with all the other support I've received on DataTables proper, I appreciate your willingness to help with a plain Javascript/jQuery question!