How to get data from populated table?
How to get data from populated table?
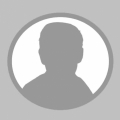
Hello,
How can I grab data from an already populated table? I don't want to select anything yet, just to simply grab everything back as an array of arrays for each row.
I have declared a global variable let table = $('#myTableId').DataTable();
and then I initialize it as a DataTable as above later on in one of my functions.
table = $('#myTableId').DataTable({
data: myArray,
select: true,
columns: [
{title: "Name"},
{title: "Step"},
...
]
});
the data from myArray is a flattened out array of arrays as shown here in this example.
I can populate the table with my data but now I wish to simply grab it back in that flattened out form to convert it to a POST request.
How can I do this? Whenever I print out the var table it shows a humongous object with each element containing a <prototype>: []
sort of element but the data is nowhere.
In simple terms, how can I save my table to a variable to be able to retrieve the data later on? There seems to be no straight answer and I already tried to do console.log(table.rows.data().toArray());
but this doesn't work. To be honest, even if I try the table.rows.data()
I don't get my data. It's as if my table is declared empty of some sorts. This is regardless if I use let
or var
to declare my variable.
This question has an accepted answers - jump to answer
Answers
Use
table.rows().data().toArray()
instead. Note therows()
. The API isrows().api()
.Kevin
Doing
table.rows().data().toArray()
returns me an empty array.Here is most of the code. Maybe I'm missing something:
I strongly believe there's an easy solution to my problem but it escapes me.
We're happy to take a look, but as per the forum rules, please link to a test case - a test case that replicates the issue will ensure you'll get a quick and accurate response. Information on how to create a test case (if you aren't able to link to the page you are working on) is available here.
Cheers,
Colin
The
$.getJSON()
ajax request is an asynchronous operation. Theconsole.log(table.rows().data().toArray());
is executing before the request is complete. Move the console.log statement inside the$.getJSON()
function after the Datatables initialization, for example:Kevin