Basic example with AJAX not working?
Basic example with AJAX not working?

I try to test this simple AJAX-example:
https://datatables.net/examples/ajax/simple.html
After executing i only get this output:
These are my files i use (all files are stored in the same folder) -
index.html:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/1.10.25/css/jquery.dataTables.min.css">
</head>
<body>
<div class="container py-5">
<header class="text-center text-white">
<h1 class="display-4">Levermann Scores</h1>
</header>
<div class="row py-5">
<div class="col-lg-10 mx-auto">
<div class="card rounded shadow border-0">
<div class="card-body p-5 bg-white rounded">
<div class="table-responsive">
<table id="example" class="display" style="width:100%">
<thead>
<tr>
<th>Name</th>
<th>Position</th>
<th>Office</th>
<th>Extn.</th>
<th>Start date</th>
<th>Salary</th>
</tr>
</thead>
<tfoot>
<tr>
<th>Name</th>
<th>Position</th>
<th>Office</th>
<th>Extn.</th>
<th>Start date</th>
<th>Salary</th>
</tr>
</tfoot>
</table>
</div>
</div>
</div>
</div>
</div>
</div>
<script type="text/javascript" charset="utf8" src="https://code.jquery.com/jquery-3.5.1.js"></script>
<script type="text/javascript" charset="utf8" src="https://cdn.datatables.net/1.10.25/js/jquery.dataTables.min.js"></script>
<script type="text/javascript" src="mainAJAX.js"></script>
</body>
</html>
mainAJAX.js
$(document).ready(function() {
$('#example').DataTable( {
"ajax": "arrays.txt"
} );
} );
and the arrays.txt (only a part of the whole file):
{
"data": [
[
"Tiger Nixon",
"System Architect",
"Edinburgh",
"5421",
"2011/04/25",
"$320,800"
],
...
...
...
[
"Donna Snider",
"Customer Support",
"New York",
"4226",
"2011/01/25",
"$112,000"
]
]
}
Why is this not working as expected?
Thanks in advance for your support.
This discussion has been closed.
Answers
If the Datatable is stuck at "Loading.." then you likely are getting a Javascript error. Take a look at your browser/s console. Does your webserver have the file in the specified path (
"ajax": "arrays.txt"
) of theajax
option?Kevin
I got this error messages in chrome:

I have put all 3 files locally in a folder:
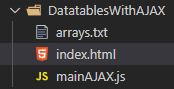
(but started no server - i thought with that command:
"ajax": "arrays.txt"
that he will access the file in the same folder structure)
for security reasons web browser's won't all ajax calls direct access to the file system. You will need a web server to send the ajax request to and the server will respond with the data. See this thread more more details.
Kevin
OK - i see - so i added a server.js (with express) to it -
server.js:
And put the arrays.txt in a data-folder like in the example:
The file-structure looks now like that:
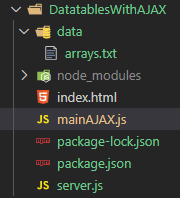
Then i started the server on the port2121

And tried to open the index.html file - but still i get the same error in the chrome browser.
You may need to do something like this:
"ajax": "localhost:2121/data/arrays.txt"
to hit the web server. Otherwise I suspect its still trying the local file system.Kevin
Hello - i tried to change the mainAJAX.js to
But i still get the error when calling the index.html in the chrome inspector:

Any ideas why this is still not working?
That's not a DataTables specific issue, it's more the case of how you're accessing the file. It would be best to search the web, as threads like this on SO are likely to point you in the right direction,
Colin
I found here another solution and added this accordingly:
https://stackoverflow.com/a/46988108/12415855
So i changed the server.js to that:
And the mainAJAX.js is still:
No i get a different error in - alert form:
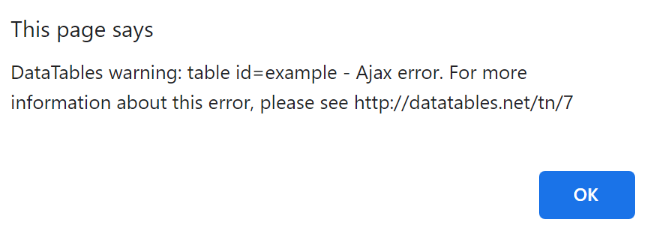
And now it shows me this error in the chrome-inspector:

Is there still something wrong with these link: http://localhost:2121/data/arrays.txt?
Beside the datatable - when i put this link (http://localhost:2121/data/arrays.txt) - should i normally see the text-file in the browser?
(cause when i do this i only get this info: Cannot GET /data/arrays.txt)
The 404 Not Found error means the file is not found in the path the web server is setup to serve files from. You will need to check this config and either change it to match the path you want to use or move the file to the path configured. You should be able to use the URL in your browser to open the file. As Colin mentioned this is outside of anything Datatables is doing. Take a look on Stack Overflow for help with setting up your web server.
Kevin
I think i found the problem -
In the server-js i have to usse express.static for the data-folder:
And in the datafolder i have to put all files (index.html, mainAJAX.js and arrays.txt).
Then i can call the text-file in the mainAJAX.js like that: