Does function called from render can be activated?
Does function called from render can be activated?
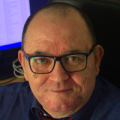
"use strict";
import {pageTitle, base_url, toastSwal} from '../common/config'
import {lang, defaultLang} from '../common/language'
import {sidebar} from '../index/sidebar'
$(document).ready( _ => {
pageTitle(lang().welcome.welcome)
$('#navbarLogout').text( lang().navbar.logout )
$('#navbarContact').text( lang().navbar.contact )
$('#usersPageTitle').text( lang().users.users )
$('#usersBreadCrumb').text( lang().users.users )
$('#usersNewUser').text( lang().users.newUser )
$('#userTable').append(
`<tfoot>
<tr>
<th>${lang().users.thId}</th>
<th>${lang().users.thFirstName}</th>
<th>${lang().users.thlastName}</th>
<th>${lang().users.thUserName}</th>
<th>${lang().users.thRoll}</th>
<th>${lang().users.thStatus}</th>
<th>${lang().users.thPosition}</th>
<th>${lang().users.thEmployeesEmail}</th>
<th>${lang().users.thCreatedAt}</th>
<th>${lang().users.thUpdatedAt}</th>
<th>${lang().users.thActions}</th>
</tr>
</tfoot>`
);
sidebar()
});
const editUser = id => {
alert( id )
return false;
}
$('#userTable').DataTable({
serverSide: false,
ajax: {
async: true,
url: 'ajaxGetAll.php',
type: 'POST',
},
columns: [
{data: "userId", title: lang().users.thId},
{data: "firstName", title: lang().users.thFirstName},
{data: "lastName", title: lang().users.thlastName},
{data: "userName", title: lang().users.thUserName},
{data: "rollName", title: lang().users.thRoll},
{data: "status", title: lang().users.thStatus},
{data: "position", title: lang().users.thPosition, defaultContent: lang().users.notAvailable},
{data: "employeesEmail", title: lang().users.thEmployeesEmail},
{data: "userCreatedAt", title: lang().users.thCreatedAt},
{data: "userUpdatedAt", title: lang().users.thUpdatedAt},
{defaultContent: '', title:lang().users.thActions, render: (data, type, row, meta) =>
{
let editButton = `<button onclick="return editUser(${row.userId});" class="btn btn-outline-primary" title="${lang().users.edit} ${row.firstName} ${row.lastName}"><i class="fa fa-edit"></i></button>`
let deleteButton = `<a type="button" onclick="return deleteUser(${row.userId})" class="btn btn-outline-danger" title="${lang().users.delete} ${row.firstName} ${row.lastName}"><i class="fa fa-trash"></i></a>`
return editButton + deleteButton;
},
},
],
columnDefs: [
{ targets: 5,
data: null,
defaultContent: '',
orderable: true,
className: 'select-checkbox'
}
],
order: [[ 0, 'desc' ]],
language: {
url: base_url('common/inc/dataTables_es_es.json'),
}
});
Error messages shown:
Uncaught ReferenceError: editUser is not defined
onclick http://localhost/helpdesk/users/:1
Description of problem:
function called from render doesn't call to it's function
This discussion has been closed.
Answers
I've never had good luck using onclick(). One thing you can try is to create an EditUser button outside of Datatables to see if it works. I don't think onclick() is a recommended way to create events. You can search Stack Overflow for more information.
The recommended way to create event handlers with Datatables is to use delegated events. See this example.
Kevin
editUser function is not in the same scope. I did solve it like this making it global.
I want to thank kthorngren for the job of answering me.
https://es.stackoverflow.com/questions/484176/error-uncaught-referenceerror-edituser-is-not-defined/484405#484405