Editor - add more attributes to option in a select list from json
Editor - add more attributes to option in a select list from json

I have a select list in Editor from a json file. I want to add more attributes to each <option> tag in the <select>. I want to read the attributes and e.g. display an alert if there is a certain text in the field "fritekst". An example would be:
<select>
<option value ="Varme 25" rel="" id="601702-601703">Varme 25</option>
<option value ="Varme 35" rel="Tom i lager. Ny leveranse ca 28.11." id="601717-601718">Varme Extreme</option>
...
</select>
The "rel" is from the "fritekst" field and the "id" is the other 2 fields combined.
So I run a check onChange() for the <select> and display the "fritekst" text.
The json (getpumplist.php):
[
{
"mod_system": "Varme 25",
"fritekst": "",
"innedel_varenr": "601702",
"utedel_varenr": "601703"
},
{
"mod_system": "Varme 35",
"fritekst": "Tom i lager. Ny leveranse ca 28.11.",
"innedel_varenr": "601717",
"utedel_varenr": "601718"
},
{
"mod_system": "Varme Extreme",
"fritekst": "Tom i lager. Ny leveranse ca 22.11",
"innedel_varenr": "601698",
"utedel_varenr": "601699"
},
{
"mod_system": "Varme Comfort",
"fritekst": "",
"innedel_varenr": "601707",
"utedel_varenr": "601708"
}
]
The field in Editor:
editor = new $.fn.dataTable.Editor( {
.........
{
type: "select",
label: "Pumpemodell",
name: "pumpemod",
value: "pumpemod",
opt: {
rel: '',
id: ''
}
},{.......
I have tried with this function to populate the select list but how do I also print the "rel=" and "id=" values from the json?
I looked into some posts about select field from json and also into the "select2" javascript but can not find out how to add the extra attributes. This code fails on the "option.attr"
var pumpmod_options = [];
$.getJSON("/teknisk/code/getpumplist.php", function(data) {
var option = {};
$.each(data, function(i,e) {
option.label = e.mod_system;
option.value = e.mod_system;
option.attr('rel', e.innedel_varenr);
pumpmod_options.push(option);
option = {};
});
}
).done(function() {
editor.field('pumpemod').update(pumpmod_options);
});
error: option.attr is not a function
Answers
Using the built-in "select" field type which is documented here:
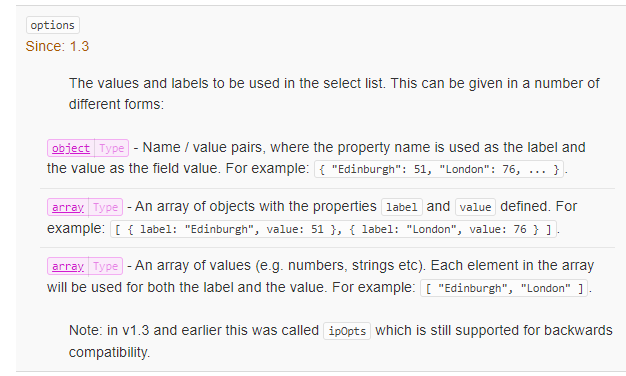
https://editor.datatables.net/reference/field/select
the options may only be the usual label - value pairs or just values.
Whether other field types allow for more you would need to find out in the respective documentation please.
https://editor.datatables.net/plug-ins/field-type/
https://editor.datatables.net/examples/plug-ins/fieldPlugin
https://editor.datatables.net/manual/development/
Just to add to that, the error is happening because you are attempting to use
option
as if it were a jQuery instance. It isn't - it's just a plain Javascript object.There is no option to set attributes for
option
tags in theselect
field type at this time. What attributes do you want to set?Allan
Thanks @rf1234 - So It seems I am not able to even use the select2 plugin for what I want? I want to add an attribute that is not label, value, placeholser or allowclear but something like "id" or "rel"but it seems impossible. At least dynamicaly? Or could I do it with a "_fieldTypes" function ?
@allan I want to set an attribute "rel=..." and "id=.." so I can use those values. The option and value does not provide enough information. I am not sure if this is a valid way to use attributes but before I put this form into dataTables I created the <select> dynamically and I could do some javascript based on those values.
What do you use them for? I'm just wondering if there is some other way of doing what your goal is. Presumably it is something client-side based on a
change
event handler? Could you look the selected value up in the list and get the attributes from there?Allan
I might have a similar use case:
- In some cases I allow the creation of new options on the fly. In that case the usual option (which is a label - value pair with "value" being the id of the selected option) does not work. In that case the "value" is the newly created option itself. On the server I realize that the sent option is not an id and I insert the new option into the database.
What does that mean for your use case?
Well you could manipulate "value" and make it contain all the attributes that you desire, e.g. as a comma separated string or whatever. Then split it up client and server side so that it works out for you.
@allan I use this for a list of products. In the product table I have e.g. name, part_number, alert_text. Under the product select is an error box. If the user chooses a product, the alert text is displayed (if exists). At the same time the part_number is written to a hidden field. This way the submitted record contains name and part_number and is alerted if the product has alert_text (like "out of stock").
@rf1234 I have thought about this approach. So I would have to filter this in the controller file? But this still does not solve the alert box with "out of stock" since that would have to pick a value from an attribute. I could of course write this to the value and parse it. Have to think about that. Seems more complicated than before when I did not use DataTables.