The example uses the rowData variable Allan used to get the row data:
function createChild_1(row, showChildren) {
//parent table data
var rowData = row.data();
var salespersonGroupingNumber = rowData.returnedSalespersonGroupNumber;
You can then do something like this:
ajax: {
type: 'POST',
url: 'BudgetReporting/getBudgetReporting_CustomerProductsData.php',
data: {salespersonGroupingNumber: salespersonGroupingNumber,
// Assumes you set the variable `customerNumber` same as `salespersonGroupingNumber` above
customerNumber: customerNumber
},
},
You need to load the Editor library. In the JS BIN page click Add Library, scroll to the bottom and select Editor. Move the added library down to load after jquery.js, etc. Like this: https://live.datatables.net/qagisada/11/edit
Exactly as you have done with your second code block there. That is making a POST Ajax call to BudgetReporting/getBudgetReporting_CustomerProductsData.php with two parameters passed to it.
That script should return a standard {"data": [...]} JSON structure, with the rows to be displayed in that data parameter.
Allan
edit Sorry - this was out of date. I was reading the end of the last page when replying!
I had to add Editor to remove the Uncaught TypeError: $.fn.dataTable.Editor is not a constructor error. Likely you will need to add select extension.
I commented out the code to initialize the child 3 Datatable and get this error:
DataTables warning: Non-table node initialisation (DIV). For more information about this error, please see http://datatables.net/tn/2
You are using the variable salespersonBillTo_childTable3 to initialize Datatables which has div elements. You need to initialize against the table within this HTML. Maybe use the table ID.
It will now give an Ajax error for ssp_BudgetData.php, but that is expected since there is no matching Ajax script on our server. It should get further on your own!
Answers
The example uses the
rowData
variable Allan used to get the row data:You can then do something like this:
Or you can skip setting the variable and do this:
Also, as Allan mentioned, you don't need to set the child table id unless you are using it for some other purpose not shown in your test cases.
Kevin
Kevin and Alan,
I got it to work based off of Alan's bin example. Your suggestion where helpful
.
Last piece of the puzzle which I trust should be a simple one for you gurus.
I also have a DataTable editor that I need to add in at the final child 3 .
When I add it in a receive error as shown in my bin example. How can I get this to work?
This was my original table look with the old script. The Next Year Budget row is the inline Editor
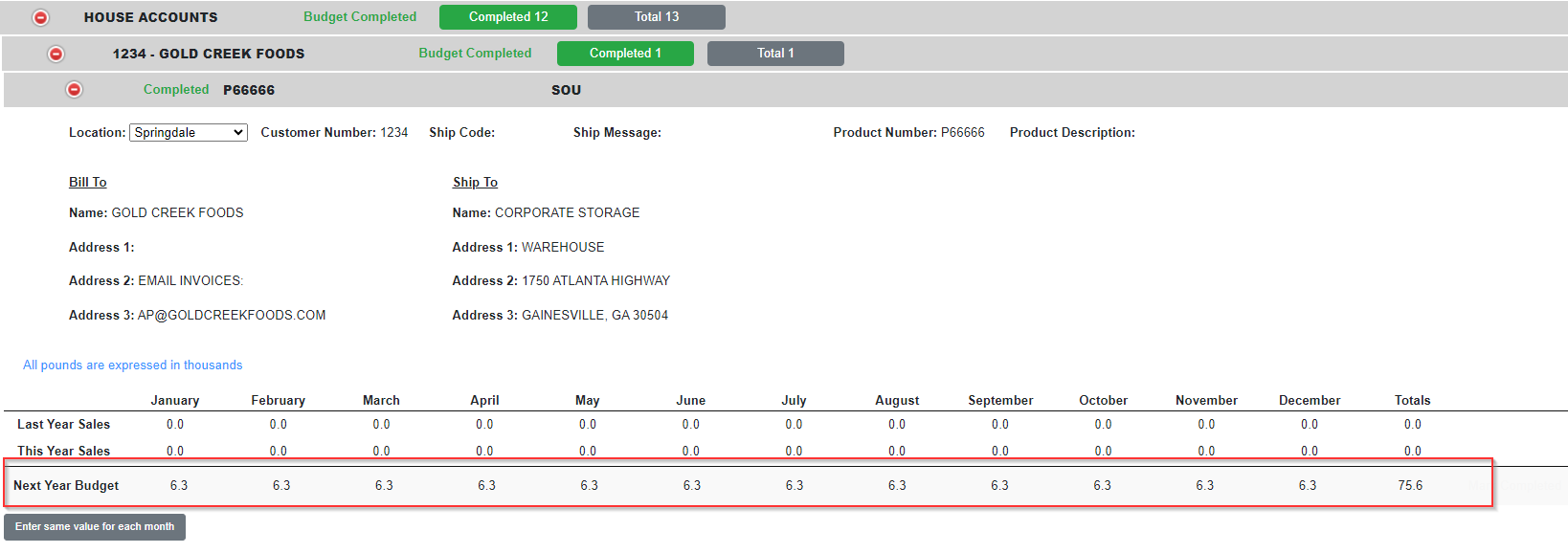
When I comment out the inline row code it works, but when I out it back in it fails .
See my latest bin. https://live.datatables.net/qagisada/10/edit
The formatting may be a little funky , because I don't have al my css in here
Obi Wan Kenobi you my only hope.
You need to load the Editor library. In the JS BIN page click Add Library, scroll to the bottom and select Editor. Move the added library down to load after jquery.js, etc. Like this:
https://live.datatables.net/qagisada/11/edit
Kevin
Exactly as you have done with your second code block there. That is making a POST Ajax call to
BudgetReporting/getBudgetReporting_CustomerProductsData.php
with two parameters passed to it.That script should return a standard
{"data": [...]}
JSON structure, with the rows to be displayed in thatdata
parameter.Allan
edit Sorry - this was out of date. I was reading the end of the last page when replying!
Kevin,
I do have those in the bin after jQuery as well is in my script in my application as well as the bin
In my previous thread I stated that it was working in my previous scripts before I changed to my script to emulated Alan's bin suggestion.
DataTable Editor inline editor row does not show up in the table
I do not get the error on my end that I see in the bin.
I really not to get this resolved today or this project is a bust.
Take a look at the test case again, all that is loaded is jquery and datatables:
https://live.datatables.net/qagisada/10/edit
I had to add Editor to remove the
Uncaught TypeError: $.fn.dataTable.Editor is not a constructor
error. Likely you will need to add select extension.I commented out the code to initialize the child 3 Datatable and get this error:
You are using the variable
salespersonBillTo_childTable3
to initialize Datatables which hasdiv
elements. You need to initialize against thetable
within this HTML. Maybe use the table ID.Kevin
I've just been experimenting with the latest bin a bit and I picked up the same issue as Kevin. I used:
to get the table you are trying to initialise as a DataTable. Updated bin: https://live.datatables.net/qagisada/13/edit .
It will now give an Ajax error for
ssp_BudgetData.php
, but that is expected since there is no matching Ajax script on our server. It should get further on your own!Allan
Thank you all . It appears all is well. Hope this is the last post in this thread..
Not to say that you may here from me on other topics.