How to use the create api post submit to create nested data?
How to use the create api post submit to create nested data?
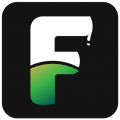
I am able to create contactInfo by editing an existing user, but i want to be able to create a user with contact info in one go. I have two mysql tables, user and contactinfo. The userid for user is auto incremented, and the id for contactInfo is autoincremented, userId is used as a foriegn key in contactInfo to link the contactInfo to the user.
( focus is on postSubmit)
userEditor.on('postSubmit', function (e, json, data, action) {
if (action === 'create') {
// Get the `userid` of the newly created user from the JSON response
var userid = 222; // Replace 'id' with the actual key for userid
console.log('New User ID:', userid);
// Create a new `contactInfo` entry using the `userid`
var contactInfoData = {
"contactInfo.userid": userid,
"contactInfo.phone": userEditor.field('contactInfo.phone').val(),
"contactInfo.email": userEditor.field('contactInfo.email').val()
};
console.log('Contact Info Data:', contactInfoData);
// Use the contactInfo editor to create the `contactInfo` entry
contactInfoEditor.create(contactInfoData, {
success: function () {
console.log('Contact Info created successfully.');
// Refresh the user table to reflect the changes
table.ajax.reload(null, true);
// Trigger the initSubmit event manually after contactInfo creation
userEditor.trigger('initSubmit');
},
error: function (xhr, err, thrown) {
console.error('Error creating contactInfo:', err);
// additional debugging for the AJAX request
console.log('AJAX Request Status:', xhr.status);
console.log('AJAX Response Text:', xhr.responseText);
}
});
}
});
Why is contactInfo not being created postSubmit? Is the way im using the create function incorrect? I've attached the full js code as a file.
This question has an accepted answers - jump to answer
Answers
There are a few errors in the code above:
create()
method doesn't accept the values for the field as the first parameter. Rather you should useval()
.success
anderror
options for the second parameter passed to thecreate()
method. It is aform-options
object.contactInfoEditor
form isn't being submitted. You need to add a call tosubmit()
.submit()
does have a callback option for success and error (first and second parameters - not in an object), so you can move that logic to there.Allan
I see, thank you.So it would be something like
Is there a recommended method to fetch the userid from the user after submission since its created via autoincrement in mysql? I'd also need to enforce my server side validation
Attached client side validation functions to check the fields before submitting, now i just need to fetch the proper userid, thank you
It should be in the
json
variable:I think should do it. Note that this would only work for single row editing / creation. That might or might not be an acceptable limitation for you. If not, then you'd need to handle it in a loop or with multi-editing.
Alla
Oh no that doesn't work, it seems i have a problem with my POST data. My post response is empty, while my GET response shows all the data. So when i run json.userid or json.data[0].userid, it remains undefined as the response is empty.
I used the generator to start the project
Im getting close, since userid is defined after the post, im attempting to fetch the userid via a get request by checking the latest username matching the username of the postSubmit.
Something like
I need to run a few more tests to ensure its working properly but it seems to be doing the job, any opinions on this method?
The response for an Editor edit request shouldn't be empty - particularly if it was a Generator based project. It should be responding to create and edit requests with data in the format described here.
Without being able to see it in action and the full server-side code, I can't know for sure why that would be. That said, your code looks fine, as long as you don't have two users submitting data at the same time
Allan
You're right, im looking into the main issue as this is a temporary fix, i did make it so it checks the username rather than just taking the last entry to lessen asynchronous issues but it'll need some further configuration to properly handle multiple users. The payload is sending proper data, the response is simply empty, and even if it wasn't, i don't think the userid will be present as its something that is set after the data is posted by the mysql database. ( auto increment ).
Thank you for your active support on this forum allan, i truly appreciate it
The should actually be okay. The way the Editor libraries we publish work is that after writing a new row to the database, they then read the data back from the db. It is done that way for exactly the reason you are seeing - auto increments. It also allows for triggers and other database formatting functions.
If you are using our PHP libraries, it should be doing all of that automatically. Something has gone wrong somewhere if that isn't the case.
Allan
Yes i used the generater to get the files and worked from there, it doesn't seem to be doing that, i kept getting empty POST responses which is why i opted to use the GET responses for now. It seems like i should start looking into the code more deeply.
Our libraries should never actually return empty. At the very least there should be a
{}
.What I would suggest doing is adding:
at the top of the server-side PHP files (i.e. where the Ajax call is made to -
table.{name}.php
- just inside the first<?php
). That will cause anything that is causing an error to print the error out - which it sounds like might be happening.Allan