Populate a text field by choosing an item from a select list?
Populate a text field by choosing an item from a select list?
So I have a user_id (Select field) and Full name (text field) in editor. I'm trying to populate the Full name based on the selected user_id.
{
label: 'Assigned Employee ID:',
name: 'user_id',
type: 'select',
}, {
label: 'Assigned Employee Name:',
name: 'assigned',
type: 'text',
readonly: true,
fieldInfo: \"This field is read only and is created when the assigned employee id is selected.\",
onChange: function ( data, type, set ) {
// get column indexes
// set data to assigned employee's full_name
return data.full_name;
},
}
I'm stuck trying to get the column index. Can anyone help? I read and re-read the columns().index() page but I'm just not getting it.
This question has accepted answers - jump to:
This discussion has been closed.
Answers
I would suggest using the
dependent()
method for this. There isn't anonChange
attribute for the text field (I guess it could be given in theattr
object, but that's not something I've tried).In the
dependent()
method it passes in the full data from the form, so you can get the user id directly from that or usefield().val()
to get the value.Allan
I should has said as well:
That's a DataTables API method - not Editor. Editor doesn't have any
columns()
methods - [itsfields()
]](https://editor.datatables.net/reference/api/).Allan
Thank you Allan. I'll check out dependent().
So I've tried this...
And also this...
But the change event does not trigger the show. I'm sure it's something simple that I'm missing or have wrong.
I also have a problem with StateSave. When I use StateSave any filter that is applied stays there. Funny thing is I didn't apply a filter. Is there a setting to not save the state of certain fields?
Hi @th3t1ck ,
Regarding
stateSave
, by default it will save everything, but you can remove (or add) other properties withstateSaveParams
,I'll leave Allan to reply to your other question
Cheers,
Colin
I don't think that is actually valid Javascript due to the second line. Perhaps you want:
Allan
Thank you Colin. A quick scan of the documentation on StateSaveParams looks like it may be what I'm looking for.
Hi Allan
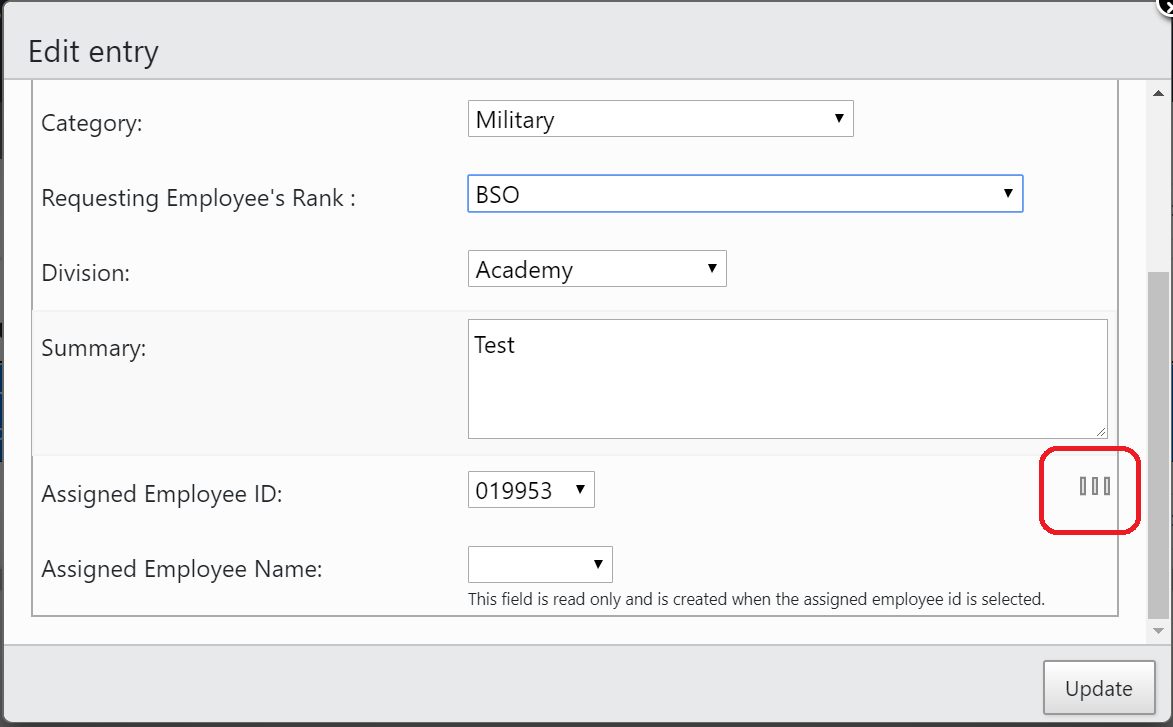
I'm not so great with Javascript as you have seen. I tried the code you suggested but it still does not work. I get this constant bar graph when the form loads. Any ideas?
Also can I have dependent() update a text field, readonly type?
Odd. Could you try this please and let me know if it works for you (i.e. no progress indicator)?
And yes,
dependent()
can manipulate any field type.Allan
Progress bar sti;; shows up with the new code.
I'm getting the following error, "Uncaught TypeError: Cannot read property 'assigned' of undefined"
At this line...
.val(data.support_requests.assigned);
Hi @th3t1ck ,
That one means that
data.support_requests
isundefined
, so can't be deferenced. Try printing it out inconsole.log()
message,Cheers,
Colin
So I used the simple example from
https://editor.datatables.net/reference/api/dependent()
and it worked fine.
I also found the the return val was actually the index/id of the user_id not the user_id itself. I still get the progress bar and the hide is not working nor is the assigned field being populated. Here is the modified code.
But it won't work, still.
Thanks for the suggestion Colin. I get the same info in the Chrome debugger's console as I received in the Network portion. I think I'm going to need to use ajax to query the database for the user_id and return the full username. I just don't know how the ajax should look like and what I should have in the php page that is called upon. I know how to query the database with php but I'm not sure how to pass the user_id to the php page and pass back the query value to the original page.
Let's aim for that then
Where
json
is the data from the server-side in the structure described independent()
to update the form.I think the key thing I missed out before (sorry!) was the
.values
from thedata
object. Forgot about that part...Allan
No problem Allan. Would the ajax file that will do the SQL query need any thing special such as json_decode, json_encode, json stringify, etc.? Do you have an example?
Allan, Colin and Kevin, Happy Holidays. Thank you for your support and patience. I greatly appreciate your help and datatables.

So I did a little work on the file called from ajax and it is looking up the user_id and getting the id (assigned field) as I am expecting. I don't know what is being passed to the find_fullname_id.php file and how to return the value that was found in the sql query back to the page calling on the ajax. What value am I doing the json_decode on?
```
<?php
//$user_id = json_decode(); // What variable goes here?
$user_id='A16479'; // manually setting variable for testing
include('../db_connect.php');
if (mysqli_connect_errno()) {
printf("Connect failed: %s\n", mysqli_connect_error());
exit();
}
$result= $dbConn->query("SELECT id FROM oesa_users WHERE user_id='A16479'");
$row_cnt = $result->num_rows;
while($row=$result->fetch_assoc()) {
$fullname_id=$row['id'];
// print'"'.$fullname_id.'", ';
}
$id = json_encode($fullname_id); // how is this value returned? What variable name should I use?
<?php > ``` ?>So I have it working the way I need it to. I found that the user_id's value was actually the row id. With that I could populate the full name in the table. Here is my code...
Thanks for your help guys.
No need for ajax or a database query since the data is already in the original ajax data returned. So simple.
Excellent - great to hear that you've got it working the way you need. Just to answer this question, if you were to use Ajax:
echo
it back - e.g.Allan
Thank you Allan. I'll probably need to call on ajax at some point and this will be good to know.