DataTables warning: table id=tblData - Invalid JSON response when using multiple include statements
DataTables warning: table id=tblData - Invalid JSON response when using multiple include statements

[HttpGet]
public IActionResult GetAll()
{
List<Location> objLocationsList = _db.Locations
.Include(l => l.Group)
.Include(l => l.County)
.ToList();
return Json(new { data = objLocationsList });
}
var dataTable;
$(document).ready(function () {
loadDataTable();
});
function loadDataTable() {
dataTable = $('#tblData').DataTable({
"responsive": true,
"ajax": { url: '/locations/getall' },
"columns": [
{ "data": "locationId", "width": "5%", "className": "dt-body-center" },
{ "data": "group", "width": "15%", "className": "dt-center" },
{ "data": "district", "width": "10%", "className": "dt-body-center" },
{ "data": "county", "width": "5%", "className": "dt-center" },
{ "data": "route", "width": "5%", "className": "dt-center" },
{ "data": "milepointBegin", "width": "5%", "className": "dt-body-center" },
{ "data": "milepointEnd", "width": "5%", "className": "dt-body-center" },
{
"data": "locationId", // Use `contactId` directly
"className": "dt-center",
"render": function (data, type, row) {
if (row.isActive) {
return `
<div class="w-75 btn-group" role="group">
<a onClick="ActiveInactive(${data}, false)" class="btn btn-success mx-2" style="cursor:pointer;">
<i class="bi bi-check-circle-fill"></i> Active
</a>
<a href="/locations/upsert?id=${data}" class="btn btn-primary mx-2">
<i class="bi bi-pencil-square"></i> Edit
</a>
<a onClick="Delete('/locations/delete/${data}')" class="btn btn-danger mx-2" style="cursor:pointer;">
<i class="bi bi-trash-fill"></i> Delete
</a>
</div>
`;
} else {
return `
<div class="w-75 btn-group" role="group">
<a onClick="ActiveInactive(${data}, true)" class="btn btn-dark text-white" style="cursor:pointer; width:100px;">
<i class="bi bi-x-circle-fill"></i> Inactive
</a>
<a href="/locations/upsert?id=${data}" class="btn btn-primary mx-2">
<i class="bi bi-pencil-square"></i> Edit
</a>
<a onClick="Delete('/locations/delete/${data}')" class="btn btn-danger mx-2" style="cursor:pointer;">
<i class="bi bi-trash-fill"></i> Delete
</a>
</div>
`;
}
},
"width": "35%"
}
]
});
}
function ActiveInactive(id, isActive) {
$.ajax({
type: "POST",
url: '/Locations/ActiveInactive',
data: JSON.stringify({ id: id, isActive: isActive }), // Pass `isActive` parameter
contentType: "application/json",
success: function (data) {
if (data.success) {
toastr.success(data.message);
dataTable.ajax.reload();
} else {
toastr.error(data.message);
}
}
});
}
function Delete(url) {
Swal.fire({
title: "Are you sure?",
text: "You won't be able to revert this!",
icon: "warning",
showCancelButton: true,
confirmButtonColor: "#3085d6",
cancelButtonColor: "#d33",
confirmButtonText: "Yes, delete it!"
}).then((result) => {
if (result.isConfirmed) {
$.ajax({
url: url,
type: 'DELETE',
success: function (data) {
dataTable.ajax.reload();
toastr.success(data.message);
}
});
}
});
}
The getall methodt works with one include statement as well as without it but not with both statements and i am not sure what is going on.
Edited by Allan - Syntax highlighting. Details on how to highlight code using markdown can be found in this guide.
This question has an accepted answers - jump to answer
Answers
I'd really need a test case showing the issue to be able to give a direct answer (which is why the forum rules state that a test case must be given).
Have you followed the instructions at the tech note that the error message links to? That would be the place to start - find out what the server is returning if it isn't valid JSON. It might give a clue as to what is going wrong.
Allan
Allan it was working yesterday morning but then it just stopped. I am not sure how to provide a test case but i did you my debugger in the browser and im not getting any errors.
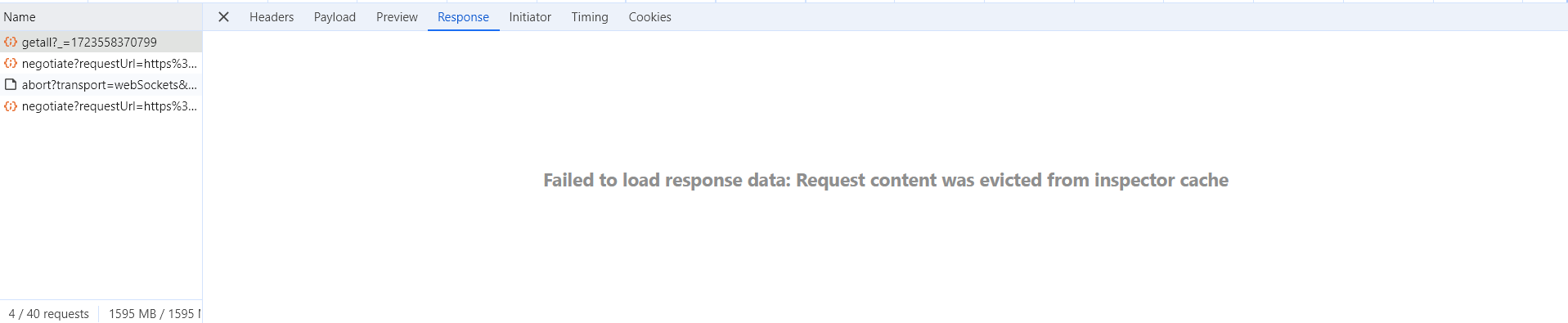

The first one -
getall
is presumably the one that has the error. But the screenshot doesn't show what the response is. Perhaps a reload and checking the response might show what it is.Allan
It will show me the results if i only use one "include" statement but when I use them both these are the results I get:
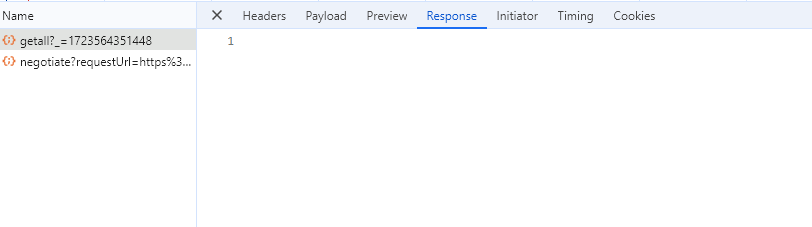

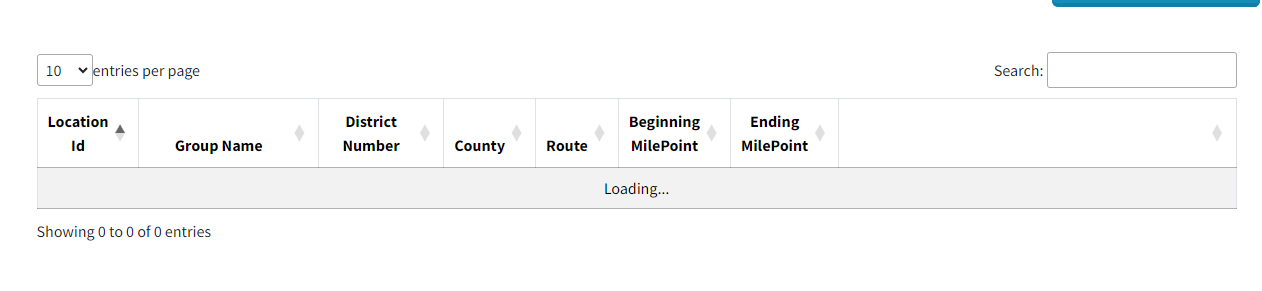
I'm getting a "200" which I know is a success message but my table is not being loaded. It's almost as if the data is getting hung up in the clouds
The empty response is not valid JSON, which is why you are getting the "Invalid JSON" error.
I'd suggest checking the server's error logs to see if there is a problem there.
Allan
I'm not familiar with your server side code but the response looks to be just the string "1". It will require debugging the server script to determine why
objLocationsList
contains only the string "1". @allan may have some ideas to help you debug. Just thought my comment might help you with a direction to start looking. Maybe there is a way to output the data query when using two includes.Kevin
I ran the application in a different browser and im still getting the "INVALID JSON" error but the response code was a little more detailed:

Next step is to follow the troubleshooting steps in the link to validate the JSON response:
https://datatables.net/manual/tech-notes/1
Kevin
@kthorngren the response contains multiple strings as show in the screenshot above. And as previously stated, if i do not use the 'include' statements the table loads and I get a response that shows the data. Same applies when i use just one include statement--either group or county--the table is still loaded and iget data in the response tab. i'm only receiving a blank response when using both statements at the same time. It worked for a minute but then bombed out and has been doing so since yesterday afternoon.
Understood. The technote links to jsonlint which is a useful tool to validate the JSON. If you are getting JSON like your last screenshoot then copy the JSON response and use jsonlint to find where the error is. If the response is blank or its invalid then the server script will need debugging.
Kevin
I'm not sure what your server environment is ( I use Python ) but maybe one of these SO threads will help.
https://stackoverflow.com/questions/45655436/json-response-gets-truncated-in-web-api-using-asp-net-core
https://stackoverflow.com/questions/60636559/json-response-breaks-in-asp-net-core-3-1-web-api-with-custom-response-wrapper/62882787#62882787
Kevin
@kthorngren I use C# but im gonna try the validation thing although i'm kinda new to this.
@kthorngren this is what i got when i copid and pasted the JSON response: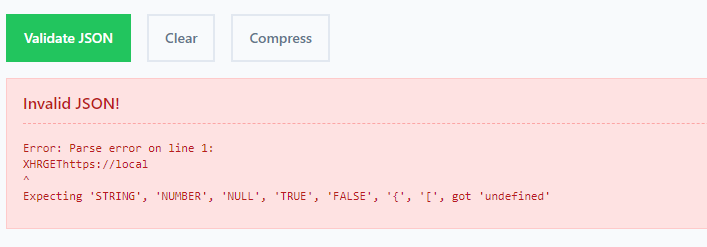
this is my controller, which alreasy contains the code to handle referenceloops:
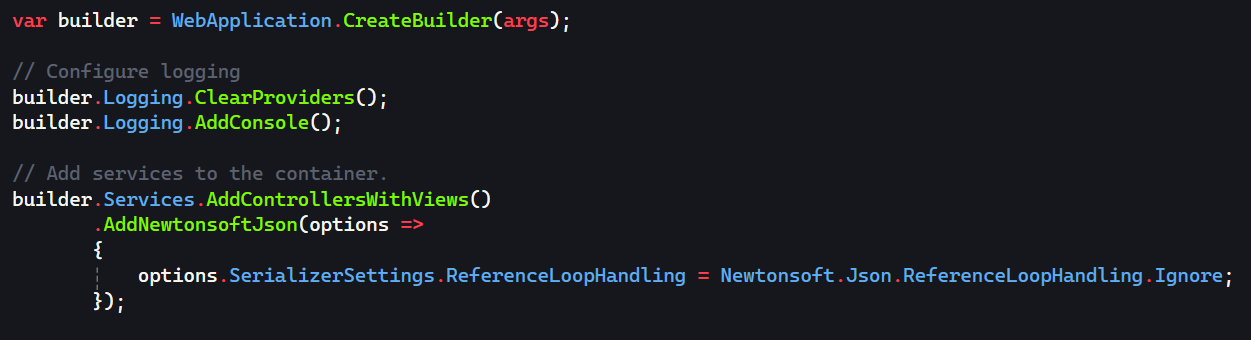
That seems odd. Not sure where you are getting the text
XHRGEThttps://local
from. I don't see that in your JSON response screeenshots. Just copy the text in theResponse
tab.Kevin
@kthorngren This is what I got when using on the code in the
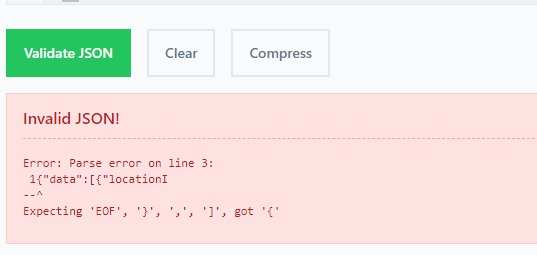
Response
tab:I'm not sure what it means tho.
Not sure why there is a leading
1
. Is that part of the text in the Response tab?I would think the
{"data":....}
would be on line 1. Are there lines before that in what you are copying or did you hit return a couple times in jsonlint?Kevin
@kthorngren, yes the

1
is a part of the text shown in theResponse
tab. See the screenshot below:I'm not sure if it is the line number or what it is but it is definitely displayed in the response code
@kthorngren I removed the
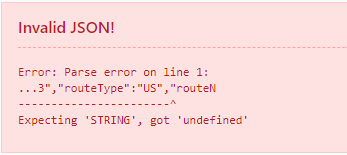
1
from the response code and only included the{"data":.....}
and this is what i got, but im still confused :Is the
1
included as part of the text of the JSON response? If so that is a problem.Its hard to say what the parse error is with just that small snippet. Can you post the full JSON response in the thread? Make sure there is no sensitive info.
Kevin
Or is
"routeN
the end of the string? Would make sense if you are seeing theResponse has been truncated
error. If so then search Stack Overflow or other C# support forum for tips on troubleshooting truncated JSON responses. Maybe one of the threads I linked will be helpful.Kevin
@kthorngren this is the full json response:
{
"data":[
{
"locationId":348,
"groupId":348,
"district":"51",
"regionId":"WEST CENTRAL",
"countyId":"38",
"routeType":"AL",
"routeNumber":"18",
"routeDescription":"3.0-4.0",
"milepointBegin":3.0,
"milepointEnd":4.0,
"adoptionBeginDate":null,
"adoptionEndDate":null,
"signShipDate":null,
"isActive":true,
"route":"AL 18",
"county":{
"countyId":"38",
"countyName":"LAMAR COUNTY",
"regionId":"WEST CENTRAL",
"locations":[
{
"locationId":352,
"groupId":352,
"district":"51",
"regionId":"WEST CENTRAL",
"countyId":"38",
"routeType":"AL",
"routeNumber":"18",
"routeDescription":"16-17",
"milepointBegin":16.0,
"milepointEnd":17.0,
"adoptionBeginDate":null,
"adoptionEndDate":null,
"signShipDate":null,
"isActive":true,
"route":"AL 18",
"group":{
"groupId":352,
"groupName":"Crossville Volunteer Fire Dept",
"groupAddress1":"1609 Highway 18",
"groupAddress2":null,
"groupCity":"Fayette",
"groupCountyId":"38",
"groupState":"AL",
"groupZip":"35555",
"groupPhone":null,
"groupEmail":null,
"groupContactName":null,
"groupNote":null,
"isActive":true,
"contacts":[
],
"locations":[
],
"visits":[
]
},
"region":null,
"visits":[
]
},
{
"locationId":355,
"groupId":355,
"district":"51",
"regionId":"WEST CENTRAL",
"countyId":"38",
"routeType":"AL",
"routeNumber":"17",
"routeDescription":"252-253",
"milepointBegin":252.0,
"milepointEnd":253.0,
"adoptionBeginDate":null,
"adoptionEndDate":null,
"signShipDate":null,
"isActive":true,
"route":"AL 17",
"group":{
"groupId":355,
"groupName":"Robert and Tommie Foster",
"groupAddress1":"54195 Highway 17",
"groupAddress2":null,
"groupCity":"Sulligent",
"groupCountyId":"38",
"groupState":"AL",
"groupZip":"35586",
"groupPhone":null,
"groupEmail":null,
"groupContactName":null,
"groupNote":null,
"isActive":true,
"contacts":[
],
"locations":[
],
"visits":[
]
},
"region":null,
"visits":[
]
},
It's not all of it because it is too long to post but this part of the original code. But again I think the
1
is just the line number:That's not going to pass jsonlint and will result in a different error because it looks like you posted just one record. Maybe you can post the record that is causing the error?
Kevin
Yes. I would think that you can't select it.
Did you see my post at 3:16?
Kevin
@kthorngren I'm assuming that
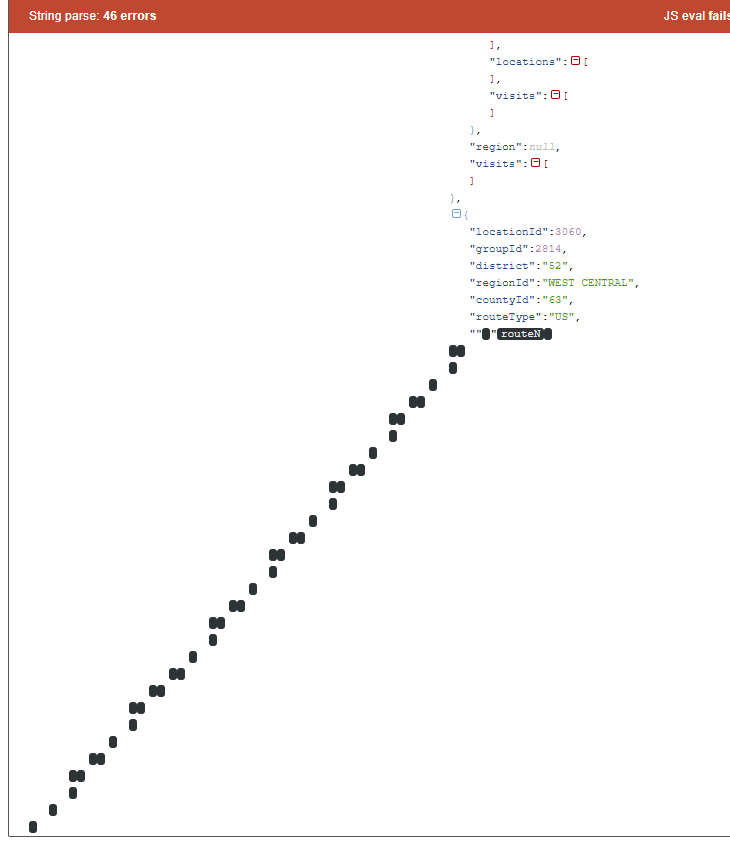
routeN
is the end of the statement based on what i saw :That looks like a corrupted JSON response. There are multiple quotes in front of
"routeNumber
and"routeNumber
is truncated with what looks like garbage following it. There isn't anything Datatables can do to fix this. The problem seems to be with the C# JSON encapsulation at the server.Kevin
@kthorngren
routeN
should actually berouteNumber
but because the response has been truncated you're not seeing the full values. Notice the beginning of the response code i posted @ 2:19. The columns are being repeated:{ "data":[ { "locationId":348, "groupId":348, "district":"51", "regionId":"WEST CENTRAL", "countyId":"38", "routeType":"AL", "routeNumber":"18", "routeDescription":"3.0-4.0", "milepointBegin":3.0, "milepointEnd":4.0, "adoptionBeginDate":null, "adoptionEndDate":null, "signShipDate":null, "isActive":true, "route":"AL 18", "county":{ "countyId":"38", "countyName":"LAMAR COUNTY", "regionId":"WEST CENTRAL", "locations":[ { "locationId":352, "groupId":352, "district":"51", "regionId":"WEST CENTRAL", "countyId":"38", "routeType":"AL", "routeNumber":"18",
Good find. Looks like your query is making a nested data structure where you might not expect that. The screenshot looks that way and this may not be fully accurate but here is the data structure of the snippet provided:
Looks like you need to debug how your data query works with two includes.
Kevin
@kthorngren i fixed it.