How to show carriage returns in the column text
How to show carriage returns in the column text

I am working on specific application that allows people to submit questions and all questions show up in the datatable and they can edit and update the question. These are stored in google sheets and I use google appscript to do the usual CRUD operations.
When people submit the question ( using bootstrap modal with "textarea" item), they add paragraphs ( line feed/carriage return). On the data table display, every thing is showing up in single line.
Actual submitted:
Actual shows up:
HTML wise: it is simple code,
<div class="container" id="dataContainer">
<br>
<div class="row">
<table id="dataT" class="table display compact" style="width: 90%">
<!-- TABLE DATA IS ADDED BY THE showData() JAVASCRIPT FUNCTION ABOVE -->
</table>
</div>
</div>
</div>
Javascript wise: simple code with button extension functionality
var table = $('#dataT').DataTable({
data: dataArray,
//CHANGE THE TABLE HEADINGS BELOW TO MATCH WITH YOUR SELECTED DATA RANGE
columns: [
{ "title": "QID" },
{ "title": "Date", "visible": false, "searchable": false },
{ "title": "Forum Name" },
..
Are there any way I can display the paragraphs instead of one line?. I removed "compact" on the table, still it shows up in the same line. as shown above "Line 1 Line 2 Line 3"
Are there any way I can display as they entered.
"Line 1
Line 2
Line 3"
I appreciate any guidance.
I will leave aside whether datatables is the right tool for this specific purpose or not. I will have to stick to it for now as I already started working on it and I am limited to tool kit I chose to work on for now as a novice to open source world.
Answers
I have one more additional question and I will put it in the same thread to have context of background.
when I initialize the table , I can access index of the data with table.rows(). But are there any I can have index what is rendered after user entered search result set. What I am trying to do is show the next question in the bootstrap modal without user going back to the datatable display.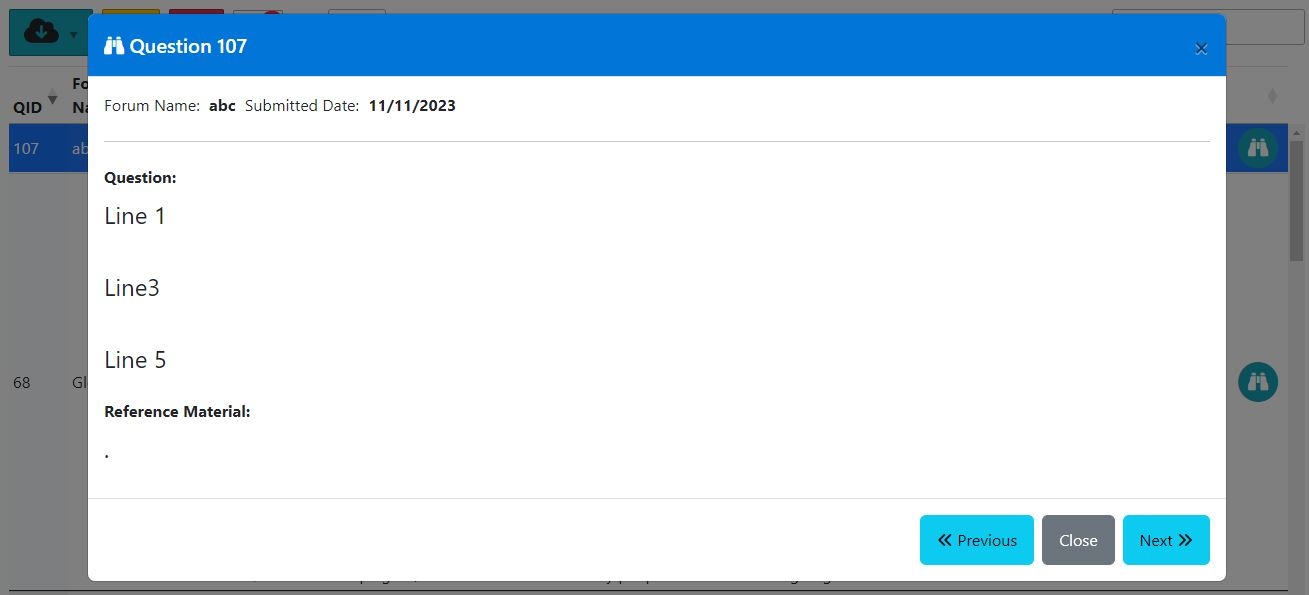
"next" and "previous" buttons should show the next row from what is displayed.
Is it possible?
I suspect the textarea field is using
\n
or\r\n
to for the newlines. You will need to convert those to an HTML break tag, ie,<br>
. Usecolumns.render
for this. For example (I didn't test this so it may need some work):See the Orthogonal data docs for details about
display
and other operations.Yes, its possible. See this Editor example. The code for this probably won't work in your solution but will need some refactoring. It relies on the Select extension extension and capabilities of using the Editor buttons to open a new editor form.
The keys are to know which row is currently being edited and how to update the Bootstrap modal with the new row. Can you provide a simple example showing what you are doing so we can offer suggestions?
https://datatables.net/manual/tech-notes/10#How-to-provide-a-test-case
Kevin
Thank you Kevin for the suggestions. I will check the options you mentioned.
Kevin Wrote:
The keys are to know which row is currently being edited and how to update the Bootstrap modal with the new row. Can you provide a simple example showing what you are doing so we can offer suggestions?
https://datatables.net/manual/tech-notes/10#How-to-provide-a-test-case
The current row being edited is identified using QID column (which is unique in the sheet/DB). I take the QID into Modal item(not displayed) when the modal is opened using java script. But to update the model with next row, I have to know the QID of the next row. If know it, I can go to sheet/database and get it and display it.
I am not sure how to get it.
In the above example current row QID is 107 ( as it is sorted descending on QID) .when I open the modal, I take the data from current row and show it in the modal. When I press "Next", I want to show the QID 68. In the database QID are sequentially stored, not the way it is showed in the current screen shot. Are there any way I can get a array of current rendered data using Javascript?
The neat thing about the example I link to is that it doesn't rely on the specific data or QID within the data to find the next row. It uses this code:
indexes
will contain therows().indexes()
of all rows in the current order of the table.currentIndex
is the index of the selected row. We will need to understand your solution better to offer suggestions of how to get thecurrentIndex
.currentPosition
is the position, within the ordered rows, of thecurrentIndex
.Next it finds either the previous or next row. This code finds the previous row:
Specifically
table.row(indexes[currentPosition - 1])
. Possibly you will need to usetable.row(indexes[currentPosition - 1]).data();
to get the row data to display in the modal.Again seeing your code will go along way for us to provide more specific suggestion. You can start with posting your Datatables init code and code to handle the modal. Better is a simple test case showing what you are doing. If you use https://live.datatables.net/ or https://jsfiddle.net/ we can update your example with code that might help.
Kevin
Thank you for suggestions.
They are helpful. I see one issue with getting the CurrentPosition. Probably I didn't understand how it works. It looks it needs some unpredictable delay to get the indexes. So I added some delay 3 seconds - some times work, some times not. Probably async/promises may be needed.
var table = $('#dataT').DataTable();
var indexes = table.rows({ search: 'applied' }).indexes();
var currentIndex = table.row({ selected: true }).index();
var currentPosition = indexes.indexOf(currentIndex);
if ( currentPosition > 0){
console.log ( ' Next: currentPosition: '+ currentPosition +'currentIndex: '+ JSON.stringify(currentIndex));
}
else{
setTimeout( function() {
$('.spinner-border').show();
var indexes = table.rows({ search: 'applied' }).indexes();
var currentIndex = table.row({ selected: true }).index();
var currentPosition = indexes.indexOf(currentIndex);
console.log ( ' After 1 sec currentPosition: '+ currentPosition +'currentIndex: '+ JSON.stringify(currentIndex));
$('.spinner-border').hide();
}, 1000 );
Here is the code https://jsfiddle.net/psign/27wm8fjn/3/
I made some changes to remove Prev button to avoid navigation confusions. I appreciate if you can suggest to make delay predictable. Thank you
Unfortunately your test case doesn't run. There is a lot of code which is difficult for us to dig through and follow. Please update the test case so it runs and shows the issue so we can do some debugging. Maybe you can simplify the code to what is needed to show the issue. Or post a link to your page.
One problem is it looks like you are loading datatables.js multiple times which can cause problems. You should load it and the extensions only once. Lines 10, 26 and 34. Looks like you are also loading bootstrap.css and fontawsome more than once. To avoid CSS conflicts I suggest loading these once.
You shouldn't need to use setTimeout. I'm not familiar with the Google API but it looks like you are using
google.script.run.withSuccessHandler()
to send data to the server. Its possible you might need to disable the prev next buttons before sending the update then in the success handler re-enable them.I would take a step back and start a new test case with some sample table data without the Google CRUD code. You probably won't need things like the export buttons etc. Just make something bare minimum to show the table and modal. Get the modal form's prev and next buttons working with the table. We can help with this.
Kevin
Thank you Kevin for the input
I had a bit of time so I built a simple example:
https://live.datatables.net/riwiyuza/1/edit
This example might not reflect how you are using modals and it doesn't perform CRUD operations. Its just meant to show how to use the relevant code from the Editor Prev/Next example.
The example uses a custom button to open the modal. It uses the
selectedSingle
button to activate the button only when one row is selected. The button uses jQuery to show the modal, for example:The
shown.bs.modal
event handler calls a function that populates the modal with the selected row's data.The showRow() function gets the selected row and populated the modal with the row data. The Prev and Next buttons have event handlers to find the next or previous row, select the row then call the showRow() function to re-populate the modal form.
Hopefully this example will help integrate into your solution.
Kevin